[TOC]
# React Navigation
Doc:[https://reactnavigation.org/docs/en/navigating.html](https://reactnavigation.org/docs/en/navigating.html)
以下内容直接整理自最新版的英文文档(只有 Windows 电脑所以只调试 Android):版本 3.x,还参考了:[https://www.jianshu.com/p/e69d248f2f0f](https://www.jianshu.com/p/e69d248f2f0f)
<span style="font-size: 20px;">Installation<span>
`npm install react-navigation`
`npm install react-native-gesture-handler react-native-reanimated`
如果使用的 React Native 版本是 0.60 或者更高,那么就不需要其他操作了。
## 导航器
导航器(Navigator)可以看作是一个普通的 React 组件,可以通过导航器来定义 APP 的导航结构,导航器还可以渲染通用元素,比如配置标题栏和选项卡栏,在 React-navigation 中有一下一些创建导航器的方法:
- createStackNavigator
- createSwitchNavigator
- createDrawerNavigator
- createBottomTabNavigator
- createMaterialBottomTabNavigator
- createMaterialTopTabNavigator
<span style="font-size: 20px;">与导航器相关的属性<span>
* navigation prop(屏幕导航属性):通过 navigation 可以完成屏幕之间的调度操作
* navigationOptions(屏幕导航选项):通过 navigationOptions 可以定制导航器显示屏幕的方式(头部标题,选项卡标签等)
<span style="font-size: 20px;">基础示例<span>
```js
// In App.js in a new project
import React from "react";
import { View, Text } from "react-native";
import { createStackNavigator, createAppContainer } from "react-navigation";
class HomeScreen extends React.Component {
render() {
return (
<View style={{ flex: 1, alignItems: "center", justifyContent: "center" }}>
<Text>Home Screen</Text>
</View>
);
}
}
const AppNavigator = createStackNavigator({
Home: {
screen: HomeScreen
}
});
export default createAppContainer(AppNavigator);
```
<span style="font-size: 20px;">导航跳转<span>
`this.props.navigation`:navigation 将作为 prop 被传递给每个 Navigator 下的 screen component
`navigate('Details')`:作为 navigation 的方法,用于跳转到另一个导航组件(与 createStackNavigator 中的对应);如果传入一个未在 stack navigator 中定义的 name,那么什么都不会发生。
```js
import React from 'react';
import { Button, View, Text } from 'react-native';
import { createStackNavigator, createAppContainer } from 'react-navigation';
class HomeScreen extends React.Component {
render() {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Home Screen</Text>
<Button
title="Go to Details"
onPress={() => this.props.navigation.navigate('Details')}
/>
</View>
);
}
}
// ... other code from the previous section
```
>Let's suppose that we actually *want* to add another details screen. This is pretty common in cases where you pass in some unique data to each route (more on that later when we talk about`params`!). To do this, we can change`navigate`to`push`. This allows us to express the intent to add another route regardless of the existing navigation history.
考虑这么一种情况:我们要添加重复的 details screen(两个 detail 页),如果继续使用`navigate`方法那么什么都不会发生;所以需要用`push`方法来代替:
```js
<Button
title="Go to Details... again"
onPress={() => this.props.navigation.push('Details')}
/>
```
>Each time you call`push`we add a new route to the navigation stack. When you call`navigate`it first tries to find an existing route with that name, and only pushes a new route if there isn't yet one on the stack.
<span style="font-size: 20px;">Going Back<span>
一般手机的头部都会有一个回退按钮,如果想手动触发回退的行为可以使用`this.porps.navigation.goBack()`:
```js
class DetailsScreen extends React.Component {
render() {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Details Screen</Text>
<Button
title="Go to Details... again"
onPress={() => this.props.navigation.push('Details')}
/>
<Button
title="Go to Home"
onPress={() => this.props.navigation.navigate('Home')}
/>
<Button
title="Go back"
onPress={() => this.props.navigation.goBack()}
/>
</View>
);
}
}
```
可以在官网提供的示例进行体验:[https://snack.expo.io/@react-navigation/going-back-v3](https://snack.expo.io/@react-navigation/going-back-v3)
<span style="font-size: 20px;">小结<span>
- `this.props.navigation.navigate('RouteName')`:将一个新的路由(route)推入导航器栈(stack navigator),如果该路由已存在于栈中则什么都不做,否则跳到对应的屏幕(screen)
- `this.props.navigation.push('RouteName')`:相比于`navigate`方法,其可以无限次地推入路由(无论名称是否相同)。
- `this.props.navigation.goBack()`:如果需要手动触发回退行为,可以使用该方法
- 如果要回到一个已经存在于栈中的屏幕(screen)可以使用`this.props.navigation.navigate('RouteName')`,如果要回到栈中的第一个屏幕可以使用`this.props.navigation.popToTop()`
- The`navigation`prop is available to all screen components (components defined as screens in route configuration and rendered by React Navigation as a route).
### 导航的生命周期
Consider a stack navigator with screens A and B. After navigating to A, its`componentDidMount`is called. When pushing B, its`componentDidMount`is also called, but A remains mounted on the stack and its`componentWillUnmount`is therefore not called.
When going back from B to A,`componentWillUnmount`of B is called, but`componentDidMount`of A is not because A remained mounted the whole time.
### 传递参数
1. Pass params to a route by putting them in an object as a second parameter to the`navigation.navigate`function:`this.props.navigation.navigate('RouteName', { /* params go here */ })`
2. Read the params in your screen component:`this.props.navigation.getParam(paramName, defaultValue)`.
```js
class HomeScreen extends React.Component {
render() {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Home Screen</Text>
<Button
title="Go to Details"
onPress={() => {
/* 1. Navigate to the Details route with params */
this.props.navigation.navigate('Details', {
itemId: 86,
otherParam: 'anything you want here',
});
}}
/>
</View>
);
}
}
class DetailsScreen extends React.Component {
render() {
/* 2. Get the param, provide a fallback value if not available */
const { navigation } = this.props;
const itemId = navigation.getParam('itemId', 'NO-ID');
const otherParam = navigation.getParam('otherParam', 'some default value');
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Details Screen</Text>
<Text>itemId: {JSON.stringify(itemId)}</Text>
<Text>otherParam: {JSON.stringify(otherParam)}</Text>
<Button
title="Go to Details... again"
onPress={() =>
this.props.navigation.push('Details', {
itemId: Math.floor(Math.random() * 100),
})}
/>
<Button
title="Go to Home"
onPress={() => this.props.navigation.navigate('Home')}
/>
<Button
title="Go back"
onPress={() => this.props.navigation.goBack()}
/>
</View>
);
}
}
```
## 使用示例
1、createBottomTabNavigator(底部 Tab 导航)
```js
import React from 'react'
import { Text, View } from 'react-native'
import { createBottomTabNavigator, createAppContainer } from 'react-navigation'
class HomeScreen extends React.Component {
render () {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Home!</Text>
</View>
)
}
}
class SettingsScreen extends React.Component {
render () {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Settings!</Text>
</View>
)
}
}
const TabNavigator = createBottomTabNavigator({
Home: { screen: HomeScreen },
Settings: { screen: SettingsScreen }
})
export default createAppContainer(TabNavigator)
```
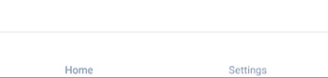
<br />
2、createMaterialBottomTabNavigator()
# react-native-vector-icons
github: [https://github.com/oblador/react-native-vector-icons](https://github.com/oblador/react-native-vector-icons)
搜索需要使用的图标:[https://oblador.github.io/react-native-vector-icons/](https://oblador.github.io/react-native-vector-icons/)
用途:在 RN 项目中使用 icon
`npm install react-native-vector-icons`
`react-native link react-native-vector-icons`:这个命令做一些与原生模块的关联?
step1:搜索以得到我们想要的图标:

step2:引入该图标所属的组件`import NavigationUtil from "../navigator/NavigationUtil";`
step3:这么使用
```js
<MaterialIcons
name={'whatshot'}
size={26}
style={{color: tintColor}}
/>
```
| Prop | Description | Default |
| --- | --- | --- |
| **`size`** | Size of the icon, can also be passed as`fontSize`in the style object. | `12` |
| **`name`** | What icon to show, see Icon Explorer app or one of the links above. | *None* |
| **`color`** | Color of the icon. | *Inherited* |
# 与 redux 集成
`npm install react-navigation-redux-helpers`
- 序言 & 更新日志
- H5
- Canvas
- 序言
- Part1-直线、矩形、多边形
- Part2-曲线图形
- Part3-线条操作
- Part4-文本操作
- Part5-图像操作
- Part6-变形操作
- Part7-像素操作
- Part8-渐变与阴影
- Part9-路径与状态
- Part10-物理动画
- Part11-边界检测
- Part12-碰撞检测
- Part13-用户交互
- Part14-高级动画
- CSS
- SCSS
- codePen
- 速查表
- 面试题
- 《CSS Secrets》
- SVG
- 移动端适配
- 滤镜(filter)的使用
- JS
- 基础概念
- 作用域、作用域链、闭包
- this
- 原型与继承
- 数组、字符串、Map、Set方法整理
- 垃圾回收机制
- DOM
- BOM
- 事件循环
- 严格模式
- 正则表达式
- ES6部分
- 设计模式
- AJAX
- 模块化
- 读冴羽博客笔记
- 第一部分总结-深入JS系列
- 第二部分总结-专题系列
- 第三部分总结-ES6系列
- 网络请求中的数据类型
- 事件
- 表单
- 函数式编程
- Tips
- JS-Coding
- Framework
- Vue
- 书写规范
- 基础
- vue-router & vuex
- 深入浅出 Vue
- 响应式原理及其他
- new Vue 发生了什么
- 组件化
- 编译流程
- Vue Router
- Vuex
- 前端路由的简单实现
- React
- 基础
- 书写规范
- Redux & react-router
- immutable.js
- CSS 管理
- React 16新特性-Fiber 与 Hook
- 《深入浅出React和Redux》笔记
- 前半部分
- 后半部分
- react-transition-group
- Vue 与 React 的对比
- 工程化与架构
- Hybird
- React Native
- 新手上路
- 内置组件
- 常用插件
- 问题记录
- Echarts
- 基础
- Electron
- 序言
- 配置 Electron 开发环境 & 基础概念
- React + TypeScript 仿 Antd
- TypeScript 基础
- 样式设计
- 组件测试
- 图标解决方案
- Algorithm
- 排序算法及常见问题
- 剑指 offer
- 动态规划
- DataStruct
- 概述
- 树
- 链表
- Network
- Performance
- Webpack
- PWA
- Browser
- Safety
- 微信小程序
- mpvue 课程实战记录
- 服务器
- 操作系统基础知识
- Linux
- Nginx
- redis
- node.js
- 基础及原生模块
- express框架
- node.js操作数据库
- 《深入浅出 node.js》笔记
- 前半部分
- 后半部分
- 数据库
- SQL
- 面试题收集
- 智力题
- 面试题精选1
- 面试题精选2
- 问答篇
- Other
- markdown 书写
- Git
- LaTex 常用命令