[TOC]
>
# 说明
因为平时经常要驱动步进电机,在这里写一个简单的驱动四相八拍电机,这个模块主要是为了配合MCU,MCU给出方向,步进步数,然后给一个上升沿的触发信号就可以了。
> # 组成模块
## 定时器模块
用于产生驱动步进电机的脉冲,可对时钟进行分频
timer:
```verilog
`timescale 1ns / 1ps
// ********************************************************************
// FileName : timer.v
// Author :hpy
// Email :yuan_hp@qq.com
// Date :2020年11月23日
// Description :一个用verilog实现的定时器
// --------------------------------------------------------------------
module timer #(parameter WIDTH = 32)(
input clk,
input rst_n,
input [ WIDTH - 1 : 0 ] T, //周期
input [ WIDTH - 1 : 0 ] duty, //占空比
output reg[ 0 : 0 ] intr , //中断信号
output pwm //输出pwm
);
reg[ WIDTH - 1 : 0 ] Cnt;
reg[ 0 : 0 ] pwm_reg;
assign pwm = (T==0)? 0 : (T == 1)? clk : pwm_reg;
always@(posedge clk or negedge rst_n)
begin
if(!rst_n)begin
Cnt <= 0;
intr <= 0;
end
else begin
if(Cnt >= T - 1'b1) begin Cnt <= 0; intr <= 1'b1; end
else begin Cnt <= Cnt + 1'b1; intr <= 1'b0; end
pwm_reg <= (Cnt<= duty)?1'b1:1'b0;
end
end
endmodule
```
## 可控脉冲生成模块
用于生成给定步数的脉冲
MultiPluseGen.v
```verilog
`timescale 1ns / 1ps
// ********************************************************************
// FileName : MultiPluseGen.v
// Author :hpy
// Email :yuan_hp@qq.com
// Date :2020年11月24日
// Description :触发一次产生多个脉冲,脉冲个数可控
// --------------------------------------------------------------------
module MultiPluseGen #(
parameter integer WIDTH = 32
)(
input clk, //
input rst_n,
input en,
input start, //触发信号标志, 上升沿触发
input [ WIDTH - 1 : 0] step,//产生的脉冲个数
input [ WIDTH - 1 : 0] period, //产生的脉冲周期,对clk的分频
output pluse,
output done //一次触发完成标志,1 触发完成 0 等待触发或者触发中
);
wire start_p ,work_clk ;
reg start_f1;
reg [ WIDTH - 1 : 0 ] cnt , step_reg ;
assign start_p = start & ~start_f1; //check posedge
assign pluse = ((cst == S1) && en )? work_clk : 1'b0;
assign done = cst == S0;
localparam S0 = 1'b0,
S1 = 1'b1;
reg [0:0] cst,nst;
always@(posedge work_clk or negedge rst_n)
begin
if(!rst_n)start_f1 <= 0;
else if(en)start_f1 <= start;
end
//go to next status
always@(posedge work_clk or negedge rst_n)
begin
if(!rst_n)begin
cst <= S0;
end
else begin
cst <= en ? nst : cst;
end
end
//
//
always@(*)
begin
//nst = S0;
case(cst)
S0: nst = (en & start_p) ? S1 : S0; // if en and check the posedge of start,go to S1 status
S1: nst = ((1==en) && (cnt>= step_reg)) ? S0:S1;
default: nst = S0;
endcase
end
always @ (posedge work_clk or negedge rst_n ) begin
if(!rst_n)begin
cnt <= 0;
step_reg <= 0;
end
else begin
if(en)begin
if((cst == S0) && (start_p==1'b1)) begin
cnt <= 1;
step_reg <= step;
end
else if(nst == S1) cnt <= cnt + 1;
else cnt <= 0;
end
end
end
wire [WIDTH - 1 : 0]duty;
assign duty = (period >> 1) - 1'b1;
wire sclk;
assign sclk = en ;
timer timer_inst (
.clk(clk),
.rst_n(rst_n),
.T(period), //周期
.duty(duty), //占空比
.pwm(work_clk)
);
endmodule
```
## 将脉冲转化为输出的模块
StepMotor.v
```verilog
`timescale 1ns / 1ps
// ********************************************************************
// FileName : StepMotor48.v
// Author :hpy
// Email :yuan_hp@qq.com
// Date :2020年11月24日
// Description :这是五线四相电机驱动模块(四象八拍电机) pwm --> 驱动
// --------------------------------------------------------------------
module StepMotor48(
input pwm,
input rst_n,
input en,
input dir,
output reg [3:0] driver
);
reg [7:0] cst, nst; // status
localparam S0 = 4'b1000,
S1 = 4'b1100,
S2 = 4'b0100,
S3 = 4'b0110,
S4 = 4'b0010,
S5 = 4'b0011,
S6 = 4'b0001,
S7 = 4'b1001;
// go to next state transition
always @(posedge pwm or negedge rst_n) begin
if(!rst_n)begin
cst = S0;
end
else begin
if(en) cst = nst;
end
end
//state transition
always @ (cst) begin
nst = S0;
case(cst)
S0: nst = en? S1 :S0 ;
S1: nst = en? S2 :S1 ;
S2: nst = en? S3 :S2 ;
S3: nst = en? S4 :S3 ;
S4: nst = en? S5 :S4 ;
S5: nst = en? S6 :S5 ;
S6: nst = en? S7 :S6 ;
S7: nst = en? S0 :S7 ;
default:nst = S0;
endcase
end
//out
integer i;
always@(posedge pwm or negedge rst_n) begin
if(! rst_n )begin
driver = S0;
end
else begin
if(en)begin
if(dir) driver = nst;
else for(i=0;i<4;i=i+1) driver[i] = nst[3-i] ;
end
end
end
endmodule
```
## 仿真时序生成
tb.v
```verilog
`timescale 1ns / 1ps
module tb ;
reg clk,rst_n;
//生成始时钟
parameter NCLK = 20; //此时时钟为50MHz
initial begin
clk=0;
forever clk=#(NCLK/2) ~clk;
end
/****************** ADD module inst ******************/
reg [31:0] step;
reg start,dir , en;
wire done, pluse;
MultiPluseGen #(.WIDTH(32)) MultiPluseGen_inst (
.clk(clk), //
.rst_n(rst_n),
.en(en),
.start(start), //触发信号标志, 上升沿触发
.step(step),//产生的脉冲个数
.period(5), //产生的脉冲周期,对clk的分频
.done(done), //一次触发完成标志,1 触发完成 0 等待触发或者触发中
.pluse(pluse)
);
StepMotor48 motor(
.pwm(pluse),
.rst_n(rst_n),
.en(en),
.dir(dir)
);
/****************** --- module inst ******************/
initial begin
$dumpfile("wave.lxt2");
$dumpvars(0, tb); //dumpvars(深度, 实例化模块1,实例化模块2,.....)
end
initial begin
rst_n = 1;
start = 0;
dir = 0;
step=0;
#(NCLK) rst_n=0;
#(NCLK) rst_n=1; //复位信号
repeat(10000) @(posedge MultiPluseGen_inst.work_clk)begin
# 1 ; //作为延时时间
if(done)begin
start = $random;
en = $random;
dir = $random;
if(start)step[3:0] = $random;
end
else start = 0;
end
$display("运行结束!");
$dumpflush;
$finish;
$stop;
end
endmodule
```
> # 仿真结果
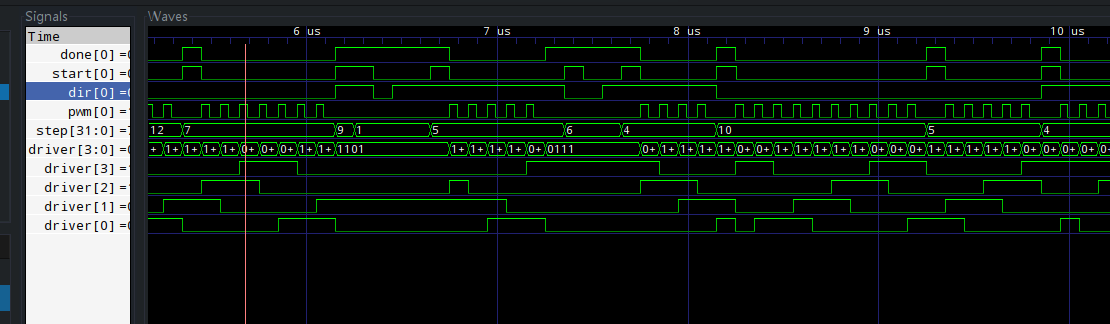
- 序
- 第1章 Linux下开发FPGA
- 1.1 Linux下安装diamond
- 1.2 使用轻量级linux仿真工具iverilog
- 1.3 使用linux shell来读写串口
- 1.4 嵌入式上的linux
- 设备数教程
- linux C 标准库文档
- linux 网络编程
- 开机启动流程
- 1.5 linux上实现与树莓派,FPGA等通信的串口脚本
- 第2章 Intel FPGA的使用
- 2.1 特别注意
- 2.2 高级应用开发流程
- 2.2.1 生成二进制bit流rbf
- 2.2.2 制作Preloader Image
- 2.2.2.1 生成BSP文件
- 2.2.2.2 编译preloader和uboot
- 2.2.2.3 更新SD的preloader和uboot
- 2.3 HPS使用
- 2.3.1 通过JTAG下载代码
- 2.3.2 HPS软件部分开发
- 2.3 quartus中IP核的使用
- 2.3.1 Intel中RS232串口IP的使用
- 2.4 一些问题的解决方法
- 2.4.1 关于引脚的复用的综合出错
- 第3章 关于C/C++的一些语法
- 3.1 C中数组作为形参不传长度
- 3.2 汇编中JUMP和CALL的区别
- 3.3 c++中map的使用
- 3.4 链表的一些应用
- 3.5 vector的使用
- 3.6 使用C实现一个简单的FIFO
- 3.6.1 循环队列
- 3.7 C语言不定长参数
- 3.8 AD采样计算同频信号的相位差
- 3.9 使用C实现栈
- 3.10 增量式PID
- 第4章 Xilinx的FPGA使用
- 4.1 Alinx使用中的一些问题及解决方法
- 4.1.1 在Genarate Bitstream时提示没有name.tcl
- 4.1.2 利用verilog求位宽
- 4.1.3 vivado中AXI写DDR说明
- 4.1.4 zynq中AXI GPIO中断问题
- 4.1.5 关于时序约束
- 4.1.6 zynq的PS端利用串口接收电脑的数据
- 4.1.7 SDK启动出错的解决方法
- 4.1.8 让工具综合是不优化某一模块的方法
- 4.1.9 固化程序(双核)
- 4.1.10 分配引脚时的问题
- 4.1.11 vivado仿真时相对文件路径的问题
- 4.2 GCC使用Attribute分配空间给变量
- 4.3 关于Zynq的DDR写入byte和word的方法
- 4.4 常用模块
- 4.4.1 I2S接收串转并
- 4.5 时钟约束
- 4.5.1 时钟约束
- 4.6 VIVADO使用
- 4.6.1 使用vivado进行仿真
- 4.7 关于PicoBlaze软核的使用
- 4.8 vivado一些IP的使用
- 4.8.1 float-point浮点单元的使用
- 4.10 zynq的双核中断
- 第5章 FPGA的那些好用的工具
- 5.1 iverilog
- 5.2 Arduino串口绘图器工具
- 5.3 LabVIEW
- 5.4 FPGA开发实用小工具
- 5.5 Linux下绘制时序图软件
- 5.6 verilog和VHDL相互转换工具
- 5.7 linux下搭建轻量易用的verilog仿真环境
- 5.8 VCS仿真verilog并查看波形
- 5.9 Verilog开源的综合工具-Yosys
- 5.10 sublim text3编辑器配置verilog编辑环境
- 5.11 在线工具
- 真值表 -> 逻辑表达式
- 5.12 Modelsim使用命令仿真
- 5.13 使用TCL实现的个人仿真脚本
- 5.14 在cygwin下使用命令行下载arduino代码到开发板
- 5.15 STM32开发
- 5.15.1 安装Atollic TrueSTUDIO for STM32
- 5.15.2 LED闪烁吧
- 5.15.3 模拟U盘
- 第6章 底层实现
- 6.1 硬件实现加法的流程
- 6.2 硬件实现乘法器
- 6.3 UART实现
- 6.3.1 通用串口发送模块
- 6.4 二进制数转BCD码
- 6.5 基本开源资源
- 6.5.1 深度资源
- 6.5.2 FreeCore资源集合
- 第7章 常用模块
- 7.1 温湿度传感器DHT11的verilog驱动
- 7.2 DAC7631驱动(verilog)
- 7.3 按键消抖
- 7.4 小脚丫数码管显示
- 7.5 verilog实现任意人数表决器
- 7.6 基本模块head.v
- 7.7 四相八拍步进电机驱动
- 7.8 单片机部分
- 7.8.1 I2C OLED驱动
- 第8章 verilog 扫盲区
- 8.1 时序电路中数据的读写
- 8.2 从RTL角度来看verilog中=和<=的区别
- 8.3 case和casez的区别
- 8.4 关于参数的传递与读取(paramter)
- 8.5 关于符号优先级
- 第9章 verilog中的一些语法使用
- 9.1 可综合的repeat
- 第10章 system verilog
- 10.1 简介
- 10.2 推荐demo学习网址
- 10.3 VCS在linux上环境的搭建
- 10.4 deepin15.11(linux)下搭建system verilog的vcs仿真环境
- 10.5 linux上使用vcs写的脚本仿真管理
- 10.6 system verilog基本语法
- 10.6.1 数据类型
- 10.6.2 枚举与字符串
- 第11章 tcl/tk的使用
- 11.1 使用Tcl/Tk
- 11.2 tcl基本语法教程
- 11.3 Tk的基本语法
- 11.3.1 建立按钮
- 11.3.2 复选框
- 11.3.3 单选框
- 11.3.4 标签
- 11.3.5 建立信息
- 11.3.6 建立输入框
- 11.3.7 旋转框
- 11.3.8 框架
- 11.3.9 标签框架
- 11.3.10 将窗口小部件分配到框架/标签框架
- 11.3.11 建立新的上层窗口
- 11.3.12 建立菜单
- 11.3.13 上层窗口建立菜单
- 11.3.14 建立滚动条
- 11.4 窗口管理器
- 11.5 一些学习的脚本
- 11.6 一些常用的操作语法实现
- 11.6.1 删除同一后缀的文件
- 11.7 在Lattice的Diamond中使用tcl
- 第12章 FPGA的重要知识
- 12.1 面积与速度的平衡与互换
- 12.2 硬件原则
- 12.3 系统原则
- 12.4 同步设计原则
- 12.5 乒乓操作
- 12.6 串并转换设计技巧
- 12.7 流水线操作设计思想
- 12.8 数据接口的同步方法
- 第13章 小项目
- 13.1 数字滤波器
- 13.2 FIFO
- 13.3 一个精简的CPU( mini-mcu )
- 13.3.1 基本功能实现
- 13.3.2 中断添加
- 13.3.3 使用中断实现流水灯(实际硬件验证)
- 13.3.4 综合一点的应用示例
- 13.4.5 使用flex开发汇编编译器
- 13.4.5 linux--Flex and Bison
- 13.4 有符号数转单精度浮点数
- 13.5 串口调试FPGA模板