# 1. 前言
这里记录一下这里使用`RecyclerView`的过程。
# 2. 使用
首先定义好对应子项的`xml`布局文件,这里命名为`choose_item_layout.xml`:
~~~
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
tools:ignore="RtlSymmetry"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:background="@drawable/choose_item_border_radius"
android:orientation="horizontal"
android:paddingEnd="10dp">
<RelativeLayout
tools:ignore="UselessParent"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_vertical"
android:padding="10dp">
<RadioButton
android:id="@+id/choose_category_page_item_rb"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerVertical="true"/>
<ImageView
android:id="@+id/choose_category_page_item_iv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerVertical="true"
android:layout_toEndOf="@id/choose_category_page_item_rb"
android:src="@drawable/tab_me" />
<TextView
android:id="@+id/choose_category_page_item_tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerVertical="true"
android:layout_marginStart="10dp"
android:layout_toEndOf="@id/choose_category_page_item_iv"
android:gravity="center"
android:text="@string/title"
android:textColor="@color/black"
android:textSize="18sp"/>
</RelativeLayout>
</LinearLayout>
~~~
也就是:
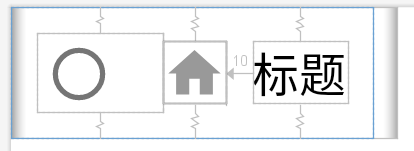
然后,在主布局文件中加入`RecyclerView`,即:
~~~
<androidx.recyclerview.widget.RecyclerView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/choose_category_page_rv"
>
</androidx.recyclerview.widget.RecyclerView>
~~~
然后为这个`RecyclerView`创建适配器:
~~~
class ChooseCategoryPageRVAdapter(context: Context, datas: MutableList<ChooseCategoryItemBean>) :
RecyclerView.Adapter<RecyclerView.ViewHolder>() {
// 列表项数据
var mItems: MutableList<ChooseCategoryItemBean> = datas
var mCtx: Context? = context
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): RecyclerView.ViewHolder {
// 实例化item
val inflateView = LayoutInflater.from(mCtx).inflate(R.layout.choose_item_layout, parent, false)
return MyViewHolder(inflateView)
}
override fun onBindViewHolder(holder: RecyclerView.ViewHolder, position: Int) {
// 设置数据
val itemView = holder as MyViewHolder
val itemData = mItems.get(position)
itemView.radioButton.isChecked = itemData.isChoose
itemView.imageView.setImageResource(itemData.img)
itemView.textView.text = itemData.text
}
override fun getItemCount(): Int {
return mItems.size
}
class MyViewHolder(itemView: View): RecyclerView.ViewHolder(itemView) {
var radioButton: RadioButton = itemView.findViewById(R.id.choose_category_page_item_rb)
var imageView: ImageView = itemView.findViewById(R.id.choose_category_page_item_iv)
var textView: TextView = itemView.findViewById(R.id.choose_category_page_item_tv)
}
}
~~~
当然,对应的`ChooseCategoryItemBean`:
~~~
data class ChooseCategoryItemBean(
var img: Int,
var text: String,
var isChoose: Boolean
)
~~~
因为这里我需要将数据存储在用户本地数据库中,所以这里需要查询数据库,至于`Room`框架的使用,这里不再介绍。直接给出使用部分:
~~~
class ChooseCategoryActivity : AppCompatActivity() {
// 找到这个RV
val choose_category_page_rv by lazy { findViewById<RecyclerView>(R.id.choose_category_page_rv) }
var chooseCategoryPageRVAdapter: ChooseCategoryPageRVAdapter? = null
val chooseCategoryItemDao: ChooseCategoryItemDao? by lazy {
MFNoteDataBase.getInstance(this)?.chooseCategoryItemDao()
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_choose_category)
// 设置数据
setDataToRecyclerView()
}
fun setDataToRecyclerView() {
// 指定为网格布局,每行放三个
val gridLayoutManager = GridLayoutManager(applicationContext, 2)
// 设置布局管理器
choose_category_page_rv.layoutManager = gridLayoutManager;
// 设置分割线,即间距
choose_category_page_rv.addItemDecoration(object : RecyclerView.ItemDecoration() {
override fun getItemOffsets(
outRect: Rect,
view: View,
parent: RecyclerView,
state: RecyclerView.State
) {
super.getItemOffsets(outRect, view, parent, state)
// 设置left/top/right/bottom的间距
outRect.set(10, 10, 10, 10)
}
})
// 加载数据
val datas: List<ChooseCategoryItemBean>? = loadItemData()
chooseCategoryPageRVAdapter =
ChooseCategoryPageRVAdapter(this, datas ?: initializationChooseCategoryItemData());
choose_category_page_rv.setAdapter(chooseCategoryPageRVAdapter);
}
fun loadItemData(): List<ChooseCategoryItemBean>? {
chooseCategoryItemDao?.apply {
return getAllChooseCategoryItem()
}
return null
}
fun initializationChooseCategoryItemData(): List<ChooseCategoryItemBean> {
val bean1 = ChooseCategoryItemBean(0, R.drawable.ic_baseline_work_24, "工作", false)
val bean5 = ChooseCategoryItemBean(4, R.drawable.ic_baseline_brush_24, "学习", false)
val bean2 = ChooseCategoryItemBean(1, R.drawable.ic_baseline_add_shopping_cart_24, "购物", false)
val bean3 = ChooseCategoryItemBean(2, R.drawable.ic_baseline_camera_roll_24, "旅游", false)
val bean4 = ChooseCategoryItemBean(3, R.drawable.ic_baseline_attach_file_24, "收藏", false)
val bean6 = ChooseCategoryItemBean(5, R.drawable.ic_baseline_directions_run_24, "运动", false)
val items = listOf(bean1, bean5, bean2, bean3, bean4, bean6)
// 存储到数据库
storageDefaultCategoryItems(items)
return items
}
// 存储默认的分类到数据库
fun storageDefaultCategoryItems(items: List<ChooseCategoryItemBean>){
for(item in items){
chooseCategoryItemDao?.insertDefault(item)
}
}
}
~~~
效果:
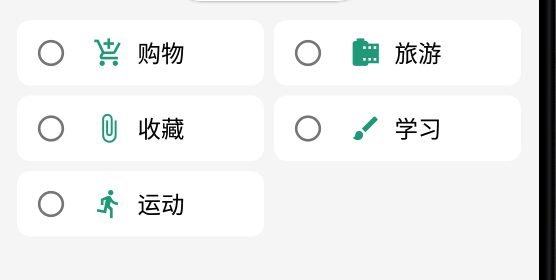
- 介绍
- UI
- MaterialButton
- MaterialButtonToggleGroup
- 字体相关设置
- Material Design
- Toolbar
- 下拉刷新
- 可折叠式标题栏
- 悬浮按钮
- 滑动菜单DrawerLayout
- NavigationView
- 可交互提示
- CoordinatorLayout
- 卡片式布局
- 搜索框SearchView
- 自定义View
- 简单封装单选
- RecyclerView
- xml设置点击样式
- adb
- 连接真机
- 小技巧
- 通过字符串ID获取资源
- 自定义View组件
- 使用系统控件重新组合
- 旋转菜单
- 轮播图
- 下拉输入框
- 自定义VIew
- 图片组合的开关按钮
- 自定义ViewPager
- 联系人快速索引案例
- 使用ListView定义侧滑菜单
- 下拉粘黏效果
- 滑动冲突
- 滑动冲突之非同向冲突
- onMeasure
- 绘制字体
- 设置画笔Paint
- 贝赛尔曲线
- Invalidate和PostInvalidate
- super.onTouchEvent(event)?
- setShadowLayer与阴影效果
- Shader
- ImageView的scaleType属性
- 渐变
- LinearGradient
- 图像混合模式
- PorterDuffXfermode
- 橡皮擦效果
- Matrix
- 离屏绘制
- Canvas和图层
- Canvas简介
- Canvas中常用操作总结
- Shape
- 圆角属性
- Android常见动画
- Android动画简介
- View动画
- 自定义View动画
- View动画的特殊使用场景
- LayoutAnimation
- Activity的切换转场效果
- 属性动画
- 帧动画
- 属性动画监听
- 插值器和估值器
- 工具
- dp和px的转换
- 获取屏幕宽高
- JNI
- javah命令
- C和Java相互调用
- WebView
- Android Studio快捷键
- Bitmap和Drawable图像
- Bitmap简要介绍
- 图片缩放和裁剪效果
- 创建指定颜色的Bitmap图像
- Gradle本地仓库
- Gradle小技巧
- RxJava+Okhttp+Retrofit构建网络模块
- 服务器相关配置
- node环境配置
- 3D特效