Fetch和Axios请求默认是不带cookie的
PS:感觉cookie使用的越来越少了,用户认证有token方案,本地存储有webStorage方案
[TOC]
## [Fetch API](https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API)
The Fetch API provides an interface for fetching resources (including across the network). It will seem familiar to anyone who has used [`XMLHttpRequest`](https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest "Use XMLHttpRequest (XHR) objects to interact with servers. You can retrieve data from a URL without having to do a full page refresh. This enables a Web page to update just part of a page without disrupting what the user is doing."), but the new API provides a more powerful and flexible feature set.
* provides a generic definition of [`Request`](https://developer.mozilla.org/en-US/docs/Web/API/Request "The Request interface of the Fetch API represents a resource request.") and [`Response`](https://developer.mozilla.org/en-US/docs/Web/API/Response "The Response interface of the Fetch API represents the response to a request.") objects (and other things involved with network requests).
* provides a definition for related concepts such as CORS and the HTTP origin header semantics, supplanting their separate definitions elsewhere.
### [Basic concepts](https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API/Basic_concepts)
At the heart of Fetch are the Interface abstractions of HTTP [`Request`](https://developer.mozilla.org/en-US/docs/Web/API/Request "The Request interface of the Fetch API represents a resource request.")s, [`Response`](https://developer.mozilla.org/en-US/docs/Web/API/Response "The Response interface of the Fetch API represents the response to a request.")s, [`Headers`](https://developer.mozilla.org/en-US/docs/Web/API/Headers "The Headers interface of the Fetch API allows you to perform various actions on HTTP request and response headers. These actions include retrieving, setting, adding to, and removing. A Headers object has an associated header list, which is initially empty and consists of zero or more name and value pairs. You can add to this using methods like append() (see Examples.) In all methods of this interface, header names are matched by case-insensitive byte sequence."), and [`Body`](https://developer.mozilla.org/en-US/docs/Web/API/Body "The Body mixin of the Fetch API represents the body of the response/request, allowing you to declare what its content type is and how it should be handled.") payloads, along with a [`global fetch`](https://developer.mozilla.org/en-US/docs/Web/API/GlobalFetch/fetch "The documentation about this has not yet been written; please consider contributing!") method for initiating asynchronous resource requests. Because the main components of HTTP are abstracted as JavaScript objects, it is easy for other APIs to make use of such functionality.
[Service Workers](https://developer.mozilla.org/en-US/docs/Web/API/ServiceWorker_API) is an example of an API that makes heavy use of Fetch.
Fetch takes the asynchronous nature of such requests one step further. The API is completely [`Promise`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise "The Promise object represents the eventual completion (or failure) of an asynchronous operation, and its resulting value.")\-based.
### Interfaces
#### 1. `Body`
[[MDN-官方文档]](https://developer.mozilla.org/en-US/docs/Web/API/Body)
Provides methods relating to the body of the response/request, allowing you to declare what its content type is and how it should be handled.
#### 2. `Headers`
[[MDN-官方文档]](https://developer.mozilla.org/en-US/docs/Web/API/Headers)
Represents response/request headers, allowing you to query them and take different actions depending on the results.
The **`Headers`** interface of the `Fetch API` allows you to perform various actions on `HTTP` request and response headers. These actions include retrieving, setting, adding to, and removing.
A`Headers`object has an associated header list, which is initially empty and consists of zero or more name and value pairs. You can add to this using methods like `append()`.
In all methods of this interface, header names are matched by case-insensitive byte sequence.
You can retrieve a `Headers` object via the `Request.headers` and `Response.headers` properties, and create a new `Headers` object using the `Headers.Headers()` constructor.
An object implementing `Headers` can directly be used in a `for...of` structure, instead of `entries()`: `for (var p of myHeaders)` is equivalent to `for (var p of myHeaders.entries())`.
>[warning] **Note**: you can find more out about the available headers by reading our [HTTP headers](https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers) reference.
**Constructor**
`Headers()`
Creates a new`Headers`object.
**Methods**
- `Headers.append()`
Appends a new value onto an existing header inside a`Headers`object, or adds the header if it does not already exist.
- `Headers.delete()`
Deletes a header from a`Headers`object.
- `Headers.entries()`
Returns an `iterator` allowing to go through all key/value pairs contained in this object.
- `Headers.forEach()`
Executes a provided function once for each array element.
- `Headers.get()`
Returns a `ByteString` sequence of all the values of a header within a`Headers`object with a given name.
- `Headers.has()`
Returns a boolean stating whether a`Headers`object contains a certain header.
- `Headers.keys()`
Returns an `iterator` allowing you to go through all keys of the key/value pairs contained in this object.
- `Headers.set()`
Sets a new value for an existing header inside a`Headers`object, or adds the header if it does not already exist.
- `Headers.values()`
Returns an `iterator` allowing you to go through all values of the key/value pairs contained in this object.
Examples
~~~javascript
var myHeaders = new Headers();
// using an append() method
myHeaders.append('Content-Type', 'text/xml');
// or, using an object:
var myHeaders = new Headers({
'Content-Type': 'text/xml'
});
// or, using an array of arrays:
var myHeaders = new Headers([
['Content-Type', 'text/xml']
]);
myHeaders.get('Content-Type') // should return 'text/xml'
~~~
#### 3. `Request`
[[MDN-官方文档]](https://developer.mozilla.org/en-US/docs/Web/API/Request)
Represents a resource request.
You can create a new `Request` object using the `Request()` constructor, but you are more likely to encounter a `Request` object being returned as the result of another API operation, such as a service worker [`FetchEvent.request`](https://developer.mozilla.org/en-US/docs/Web/API/FetchEvent/request "The request read-only property of the FetchEvent interface returns the Request that triggered the event handler.").
**Constructor**
`Request()`
Creates a new`Request`object.
**Properties**
常用
- `Request.url` | Read only
Contains the URL of the request.
- `Request.method` | Read only
Contains the request's method (`GET`,`POST`, etc.)
- `Request.mode` | Read only
Contains the mode of the request (e.g.,`cors`,`no-cors`,`same-origin`,`navigate`.)
- `Request.headers` | Read only
Contains the associated `Headers` object of the request.
- `Request.credentials` | Read only
Contains the credentials of the request (e.g.,`omit`,`same-origin`,`include`). The default is`same-origin`.
不常用
- `Request.cache` | Read only
Contains the cache mode of the request (e.g.,`default`,`reload`,`no-cache`).
- `Request.context` | Read only
Contains the context of the request (e.g.,`audio`,`image`,`iframe`, etc.)
- `Request.destination` | Read only
Returns a string from the `RequestDestination` enum describing the request's destination. This is a string indicating the type of content being requested.
- `Request.integrity` | Read only
Contains the subresource integrity value of the request (e.g.,`sha256-BpfBw7ivV8q2jLiT13fxDYAe2tJllusRSZ273h2nFSE=`).
- `Request.redirect` | Read only
Contains the mode for how redirects are handled. It may be one of`follow`,`error`, or`manual`.
- `Request.referrer` | Read only
Contains the referrer of the request (e.g.,`client`).
- `Request.referrerPolicy` | Read only
Contains the referrer policy of the request (e.g.,`no-referrer`).
`Request`implements `Body`, so it also inherits the following properties:
`body` | Read only
A simple getter used to expose a[`ReadableStream`](https://developer.mozilla.org/en-US/docs/Web/API/ReadableStream "The ReadableStream interface of the Streams API represents a readable stream of byte data. The Fetch API offers a concrete instance of a ReadableStream through the body property of a Response object.")of the body contents.
`bodyUsed` | Read only
Stores a `Boolean` that declares whether the body has been used in a response yet.
**Methods**
- `Request.clone()`
Creates a copy of the current`Request`object.
`Request` implements `Body`,so it also has the following methods available to it:
- `Body.arrayBuffer()`
Returns a promise that resolves with an[`ArrayBuffer`](https://developer.mozilla.org/en-US/docs/Web/API/ArrayBuffer "The documentation about this has not yet been written; please consider contributing!")representation of the request body.
- `Body.blob()`
Returns a promise that resolves with a `Blob` representation of the request body.
A Blob object represents a file-like object of immutable, raw data; they can be read as text or binary data, or converted into a `ReadableStream` so its methods can be used for processing the data.
Blobs can represent data that isn't necessarily in a JavaScript-native format.
The File interface is based on Blob, inheriting blob functionality and expanding it to support files on the user's system.
- `Body.formData()`
Returns a promise that resolves with a `FormData` representation of the request body.
The `formData()` method of the `Body` mixin takes a Response stream and reads it to completion. It returns a promise that resolves with a `FormData` object.
The `FormData` interface provides a way to easily construct a set of key/value pairs representing form fields and their values, which can then be easily sent using the `XMLHttpRequest.send()` method.
It uses the same format a form would use if the encoding type were set to "multipart/form-data".
- `Body.json()`
Returns a promise that resolves with a`JSON`representation of the request body.
The `json()` method of the Body mixin takes a Response stream and reads it to completion.
It returns a promise that resolves with the result of parsing the body text as JSON.
- `Body.text()`
Returns a promise that resolves with an `USVString` (text) representation of the request body..
The `text()` method of the `Body` mixin takes a Response stream and reads it to completion. It returns a promise that resolves with a USVString object (text). The response is always decoded using UTF-8.
`USVString` corresponds to the set of all possible sequences of unicode scalar values.
`USVString` maps to a `String` when returned in JavaScript; it's generally only used for APIs that perform text processing and need a string of unicode scalar values to operate on.
`USVString` is equivalent to `DOMString` except for not allowing unpaired surrogate codepoints. Unpaired surrogate codepoints present in `USVString` are converted by the browser to Unicode 'replacement character' U+FFFD, (�).
Examples:
~~~javascript
const request = new Request('https://example.com', {method: 'POST', body: '{"foo": "bar"}'});
const URL = request.url;
const method = request.method;
const credentials = request.credentials;
const bodyUsed = request.bodyUsed;
fetch(request)
.then(response => {
if (response.status === 200) {
return response.json();
} else {
throw new Error('Something went wrong on api server!');
}
})
.then(response => {
console.debug(response);
// ...
}).catch(error => {
console.error(error);
});
~~~
>[warning] **Note:** The body type can only be a `Blob`, `BufferSource`, `FormData`, `URLSearchParams`, `USVString` or `ReadableStream` type, so for adding a JSON object to the payload you need to stringify that object.
#### 4. `Response`
[[MDN-官方文档]](https://developer.mozilla.org/en-US/docs/Web/API/Response)
Represents the response to a request.
[[MDN Response]](https://developer.mozilla.org/en-US/docs/Web/API/Response)
The **`Response`** interface of the [Fetch API](https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API) represents the response to a request.
The `fetch()` call returns a promise, which resolves with the `Response` object associated with the resource fetch operation.
- **Constructor**
`Response()`: Creates a new `Response` object.
- **Properties**
| 序号 | 表达 | 说明 |
| --- | --- | --- |
| 1. | `Response.headers` | `Read only`, <br>Contains the `Headers` object associated with the response.|
| 2. | `Response.ok`| `Read only`, <br>Contains a boolean stating whether the response was successful (status in the range 200-299) or not. |
| 3. | `Response.redirected` | `Read only`, <br>Indicates whether or not the response is the result of a redirect; that is, its URL list has more than one entry. |
| 4. | `Response.status` | `Read only`, <br>Contains the status code of the response (e.g., `200` for a success). |
| 5. | `Response.statusText` | `Read only`, <br>Contains the status message corresponding to the status code (e.g., `OK` for `200`). |
| 6. | `Response.type` | `Read only`, <br>Contains the type of the response (e.g., `basic`, `cors`). |
| 7. | `Response.url` | `Read only`, <br>Contains the URL of the response. |
| 8. | `Response.useFinalURL` | Contains a boolean stating whether this is the final URL of the response. |
`Response` implements [`Body`](https://developer.mozilla.org/en-US/docs/Web/API/Body "The Body mixin of the Fetch API represents the body of the response/request, allowing you to declare what its content type is and how it should be handled."), so it also has the following properties available to it:
[`Body.body`](https://developer.mozilla.org/en-US/docs/Web/API/Body/body "The body read-only property of the Body mixin is a simple getter used to expose a ReadableStream of the body contents.") Read only, A simple getter used to expose a [`ReadableStream`](https://developer.mozilla.org/en-US/docs/Web/API/ReadableStream "The ReadableStream interface of the Streams API represents a readable stream of byte data. The Fetch API offers a concrete instance of a ReadableStream through the body property of a Response object.") of the body contents.
[`Body.bodyUsed`](https://developer.mozilla.org/en-US/docs/Web/API/Body/bodyUsed "The bodyUsed read-only property of the Body mixin contains a Boolean that indicates whether the body has been read yet.") Read only, Stores a [`Boolean`](https://developer.mozilla.org/en-US/docs/Web/API/Boolean "REDIRECT Boolean [en-US]") that declares whether the body has been used in a response yet.
**Methods**
| 序号 | 表达 | 说明 |
| --- | --- | --- |
| 1. | `Response.clone()` | Creates a clone of a `Response` object. |
| 2. | `Response.error()` | Returns a new `Response` object associated with a network error. |
| 3. | `Response.redirect()` | Creates a new response with a different URL. |
| 4. | `Body.arrayBuffer()`* | Takes a `Response` stream and reads it to completion. It returns a promise that resolves with an [`ArrayBuffer`](https://developer.mozilla.org/en-US/docs/Web/API/ArrayBuffer "The documentation about this has not yet been written; please consider contributing!"). |
| 5. | `Body.blob()`* | Takes a `Response` stream and reads it to completion. It returns a promise that resolves with a [`Blob`](https://developer.mozilla.org/en-US/docs/Web/API/Blob "A Blob object represents a file-like object of immutable, raw data. Blobs represent data that isn't necessarily in a JavaScript-native format. The File interface is based on Blob, inheriting blob functionality and expanding it to support files on the user's system."). |
| 6. | `Body.formData()`* | Takes a `Response` stream and reads it to completion. It returns a promise that resolves with a [`FormData`](https://developer.mozilla.org/en-US/docs/Web/API/FormData "The FormData interface provides a way to easily construct a set of key/value pairs representing form fields and their values, which can then be easily sent using the XMLHttpRequest.send() method. It uses the same format a form would use if the encoding type were set to "multipart/form-data".") object. |
| 7. | `Body.json()`* | Takes a `Response` stream and reads it to completion. It returns a promise that resolves with the result of parsing the body text as [`JSON`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/JSON "The JSON object contains methods for parsing JavaScript Object Notation (JSON) and converting values to JSON. It can't be called or constructed, and aside from its two method properties, it has no interesting functionality of its own."). |
| 8. | `Body.text()`* | Takes a `Response` stream and reads it to completion. It returns a promise that resolves with a [`USVString`](https://developer.mozilla.org/en-US/docs/Web/API/USVString "USVString corresponds to the set of all possible sequences of unicode scalar values. USVString maps to a String when returned in JavaScript; it's generally only used for APIs that perform text processing and need a string of unicode scalar values to operate on. USVString is equivalent to DOMString except for not allowing unpaired surrogate codepoints. Unpaired surrogate codepoints present in USVString are converted by the browser to Unicode 'replacement character' U+FFFD, (�).") (text). |
*`Response` implements `Body`, so it also has the following methods available to it:
### method
[`WindowOrWorkerGlobalScope.fetch()`](https://developer.mozilla.org/en-US/docs/Web/API/WindowOrWorkerGlobalScope/fetch)
The `fetch()` method takes one mandatory argument, the path to the resource you want to fetch. It returns a [`Promise`](https://developer.mozilla.org/en-US/docs/Web/API/Promise "REDIRECT Promise") that resolves to the [`Response`](https://developer.mozilla.org/en-US/docs/Web/API/Response "The Response interface of the Fetch API represents the response to a request.") to that request, whether it is successful or not. You can also optionally pass in an `init` options object as the second argument (see [`Request`](https://developer.mozilla.org/en-US/docs/Web/API/Request "The Request interface of the Fetch API represents a resource request."))
>[info] `fetch()`方法返回的是一个`Promise`对象,这个`Promise`对象会被resolve成一个`response`对象,该`response`对象就是对使用`fetch()`方法所发起的`request`的响应结果。
### Aborting a fetch
Browsers have started to add experimental support for the [`AbortController`](https://developer.mozilla.org/en-US/docs/Web/API/AbortController "The AbortController interface represents a controller object that allows you to abort one or more DOM requests as and when desired.") and [`AbortSignal`](https://developer.mozilla.org/en-US/docs/Web/API/AbortSignal "The AbortSignal interface represents a signal object that allows you to communicate with a DOM request (such as a Fetch) and abort it if required via an AbortController object.") interfaces (aka The Abort API), which allow operations like Fetch and XHR to be aborted if they have not already completed. See the interface pages for more details.
## Using Fetch
[[MDN Fetch]](https://developer.mozilla.org/en-US/docs/Web/API/WindowOrWorkerGlobalScope/fetch)
[[MDN Using Fetch]](https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API/Using_Fetch)
The`fetch`specification differs from`jQuery.ajax()`in two main ways:
* The Promise returned from`fetch()`**won’t reject on HTTP error status** even if the response is an HTTP 404 or 500. Instead, it will resolve normally (with`ok`status set to false), and it will only reject on network failure or if anything prevented the request from completing.
* By default,`fetch`**won't send or receive any cookies** from the server, resulting in unauthenticated requests if the site relies on maintaining a user session (to send cookies, the*credentials*[init option](https://developer.mozilla.org/en-US/docs/Web/API/WindowOrWorkerGlobalScope/fetch#Parameters)must be set).
Since [Aug 25, 2017](https://github.com/whatwg/fetch/pull/585). The spec changed the default credentials policy to`same-origin`. Firefox changed since 61.0b13.
A basic fetch request is really simple to set up. Have a look at the following code:
~~~js
fetch('http://example.com/movies.json')
.then(function(response) {
return response.json();
})
.then(function(myJson) {
console.log(JSON.stringify(myJson));
});
~~~
### Syntax
~~~js
fetchResponsePromise = fetch(resource, init);
~~~
**`resource`**
This defines the resource that you wish to fetch. This can either be:
* A[`USVString`](https://developer.mozilla.org/en-US/docs/Web/API/USVString "USVString corresponds to the set of all possible sequences of unicode scalar values. USVString maps to a String when returned in JavaScript; it's generally only used for APIs that perform text processing and need a string of unicode scalar values to operate on. USVString is equivalent to DOMString except for not allowing unpaired surrogate codepoints. Unpaired surrogate codepoints present in USVString are converted by the browser to Unicode 'replacement character' U+FFFD, (�).")containing the direct URL of the resource you want to fetch. Some browsers accept`blob:`and`data:`as schemes.
* A[`Request`](https://developer.mozilla.org/en-US/docs/Web/API/Request "The Request interface of the Fetch API represents a resource request.")object.
**`init`**(Optional)
An options object containing any custom settings that you want to apply to the request. The possible options are:
* `method`: The request method, e.g.,`GET`,`POST`. Note that the[`Origin`](https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Origin "The Origin request header indicates where a fetch originates from. It doesn't include any path information, but only the server name. It is sent with CORS requests, as well as with POST requests. It is similar to the Referer header, but, unlike this header, it doesn't disclose the whole path.")header is not set on Fetch requests with a method of`[HEAD](https://developer.mozilla.org/en-US/docs/Web/HTTP/Methods/HEAD)`or`[GET](https://developer.mozilla.org/en-US/docs/Web/HTTP/Methods/GET)`(this behavior was corrected in Firefox 65 — see[bug 1508661](https://bugzilla.mozilla.org/show_bug.cgi?id=1508661 "FIXED: origin header should not be set for GET and HEAD requests")).
* `headers`: Any headers you want to add to your request, contained within a[`Headers`](https://developer.mozilla.org/en-US/docs/Web/API/Headers "The Headers interface of the Fetch API allows you to perform various actions on HTTP request and response headers. These actions include retrieving, setting, adding to, and removing. A Headers object has an associated header list, which is initially empty and consists of zero or more name and value pairs. You can add to this using methods like append() (see Examples.) In all methods of this interface, header names are matched by case-insensitive byte sequence.")object or an object literal with[`ByteString`](https://developer.mozilla.org/en-US/docs/Web/API/ByteString "ByteString is a UTF-8 String that corresponds to the set of all possible sequences of bytes. ByteString maps to a String when returned in JavaScript; generally, it's only used when interfacing with protocols that use bytes and strings interchangably, such as HTTP.")values. Note that[some names are forbidden](https://developer.mozilla.org/en-US/docs/Glossary/Forbidden_header_name).
* `body`: Any body that you want to add to your request: this can be a[`Blob`](https://developer.mozilla.org/en-US/docs/Web/API/Blob "A Blob object represents a file-like object of immutable, raw data. Blobs represent data that isn't necessarily in a JavaScript-native format. The File interface is based on Blob, inheriting blob functionality and expanding it to support files on the user's system."),[`BufferSource`](https://developer.mozilla.org/en-US/docs/Web/API/BufferSource "BufferSource is a typedef used to represent objects that are either themselves an ArrayBuffer, or which are a TypedArray providing an ArrayBufferView."),[`FormData`](https://developer.mozilla.org/en-US/docs/Web/API/FormData "The FormData interface provides a way to easily construct a set of key/value pairs representing form fields and their values, which can then be easily sent using the XMLHttpRequest.send() method. It uses the same format a form would use if the encoding type were set to "multipart/form-data"."),[`URLSearchParams`](https://developer.mozilla.org/en-US/docs/Web/API/URLSearchParams "The URLSearchParams interface defines utility methods to work with the query string of a URL."), or[`USVString`](https://developer.mozilla.org/en-US/docs/Web/API/USVString "USVString corresponds to the set of all possible sequences of unicode scalar values. USVString maps to a String when returned in JavaScript; it's generally only used for APIs that perform text processing and need a string of unicode scalar values to operate on. USVString is equivalent to DOMString except for not allowing unpaired surrogate codepoints. Unpaired surrogate codepoints present in USVString are converted by the browser to Unicode 'replacement character' U+FFFD, (�).")object. Note that a request using the`GET`or`HEAD`method cannot have a body.
* `mode`: The mode you want to use for the request, e.g.,`cors`,`no-cors`, or`same-origin`.
* `credentials`: The request credentials you want to use for the request:`omit`,`same-origin`, or`include`. To automatically send cookies for the current domain, this option must be provided. Starting with Chrome 50, this property also takes a[`FederatedCredential`](https://developer.mozilla.org/en-US/docs/Web/API/FederatedCredential "The FederatedCredential interface of the the Credential Management API provides information about credentials from a federated identity provider. A federated identity provider is an entity that a website trusts to correctly authenticate a user, and that provides an API for that purpose. OpenID Connect is an example of a federated identity provider framework.")instance or a[`PasswordCredential`](https://developer.mozilla.org/en-US/docs/Web/API/PasswordCredential "The interface of the Credential Management API provides information about a username/password pair. In supporting browsers an instance of this class may be passed in the credential member of the init object for global fetch.")instance.
* `cache`: The[cache mode](https://developer.mozilla.org/en-US/docs/Web/API/Request/cache)you want to use for the request.
* `redirect`: The redirect mode to use:`follow`(automatically follow redirects),`error`(abort with an error if a redirect occurs), or`manual`(handle redirects manually). In Chrome the default is `follow` (before Chrome 47 it defaulted to `manual`).
* `referrer`: A[`USVString`](https://developer.mozilla.org/en-US/docs/Web/API/USVString "USVString corresponds to the set of all possible sequences of unicode scalar values. USVString maps to a String when returned in JavaScript; it's generally only used for APIs that perform text processing and need a string of unicode scalar values to operate on. USVString is equivalent to DOMString except for not allowing unpaired surrogate codepoints. Unpaired surrogate codepoints present in USVString are converted by the browser to Unicode 'replacement character' U+FFFD, (�).")specifying `no-referrer`, `client`, or a URL. The default is `client`.
* `referrerPolicy`: Specifies the value of the referer HTTP header. May be one of`no-referrer`,`no-referrer-when-downgrade`,`origin`,`origin-when-cross-origin`,`unsafe-url`.
* `integrity`: Contains the[subresource integrity](https://developer.mozilla.org/en-US/docs/Web/Security/Subresource_Integrity)value of the request (e.g.,`sha256-BpfBw7ivV8q2jLiT13fxDYAe2tJllusRSZ273h2nFSE=`).
* `keepalive`: The`keepalive`option can be used to allow the request to outlive the page. Fetch with the`keepalive`flag is a replacement for the[`Navigator.sendBeacon()`](https://developer.mozilla.org/en-US/docs/Web/API/Navigator/sendBeacon "The navigator.sendBeacon() method can be used to asynchronously transfer a small amount of data over HTTP to a web server.")API.
* `signal`: An[`AbortSignal`](https://developer.mozilla.org/en-US/docs/Web/API/AbortSignal "The AbortSignal interface represents a signal object that allows you to communicate with a DOM request (such as a Fetch) and abort it if required via an AbortController object.")object instance; allows you to communicate with a fetch request and abort it if desired via an[`AbortController`](https://developer.mozilla.org/en-US/docs/Web/API/AbortController "The AbortController interface represents a controller object that allows you to abort one or more DOM requests as and when desired.").
### Return value
A `Promise` that resolves to a `Response` objec
### Supplying request options
The`fetch()`method can optionally accept a second parameter, an`init`object that allows you to control a number of different settings:
See[`fetch()`](https://developer.mozilla.org/en-US/docs/Web/API/GlobalFetch/fetch "The documentation about this has not yet been written; please consider contributing!")for the full options available, and more details.
~~~js
// Example POST method implementation:
postData('http://example.com/answer', {answer: 42})
.then(data => console.log(JSON.stringify(data))) // JSON-string from `response.json()` call
.catch(error => console.error(error));
function postData(url = '', data = {}) {
// Default options are marked with *
return fetch(url, {
method: 'POST', // *GET, POST, PUT, DELETE, etc.
mode: 'cors', // no-cors, cors, *same-origin
cache: 'no-cache', // *default, no-cache, reload, force-cache, only-if-cached
credentials: 'same-origin', // include, *same-origin, omit
headers: {
'Content-Type': 'application/json',
// 'Content-Type': 'application/x-www-form-urlencoded',
},
redirect: 'follow', // manual, *follow, error
referrer: 'no-referrer', // no-referrer, *client
body: JSON.stringify(data), // body data type must match "Content-Type" header
})
.then(response => response.json()); // parses JSON response into native JavaScript objects
}
~~~
### CORS(Cross-Origin Resource Sharing)
[[MDN-CORS]](https://developer.mozilla.org/en-US/docs/Glossary/CORS)
Cross-Origin Resource Sharing (CORS), is a machanism/protocal/system, consisting of transmitting HTTP headers, that determines whether browsers block frontend JavaScript code from accessing responses for cross-origin requests. A web application executes a **cross-origin HTTP request** when it requests a resource that has a different origin (domain, protocol, or port) from its own.
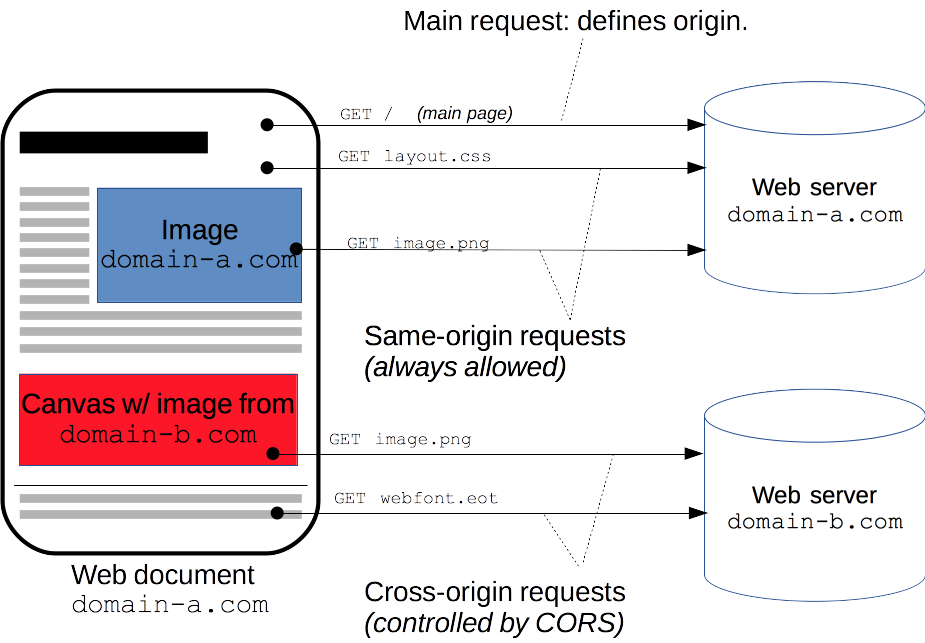
The CORS mechanism supports secure cross-origin requests and data transfers between browsers and servers. Modern browsers use CORS in a APIs such as `XMLHttpRequest` or `Fetch` to mitigate the risks of cross-origin HTTP requests.
同源策略:是指协议,域名,端口都要相同,其中有一个不同都会产生跨域;
跨域:指的是浏览器不能执行其他网站的脚本。它是由浏览器的同源策略造成的,是浏览器对javascript施加的安全限制。
例如:a页面想获取b页面资源,如果a、b页面的协议、域名、端口、子域名不同,所进行的访问行动都是跨域的,而浏览器为了安全问题一般都限制了跨域访问,也就是不允许跨域请求资源。注意:跨域限制访问,其实是**浏览器的限制**。理解这一点很重要!!!
假设有两个网站,A网站部署在:http://localhost:81 即本地ip端口81上;B网站部署在:http://localhost:82 即本地ip端口82上。现在A网站的页面想去访问B网站的信息,就会出现跨域问题。
用nginx作为代理服务器和用户交互,这样用户就只需要在80端口上进行交互就可以了,这样就避免了跨域问题,因为我们都是在80端口上进行交互的;
下面我们看一下利用nginx作为反向代理的具体配置:
> server {
>
> listen 80; #监听80端口,可以改成其他端口
>
> server\_name localhost; # 当前服务的域名
>
> #charset koi8-r;
>
> #access\_log logs/host.access.log main;
>
> location / {
>
> proxy\_pass http://localhost:81;
>
> proxy\_redirect default;
>
> }
>
> location /apis { #添加访问目录为/apis的代理配置
>
> rewrite ^/apis/(.\*)$ /$1 break;
>
> proxy\_pass http://localhost:82;
>
> }
>
> #以下配置省略
1.当用户发送localhost:80/时会被nginx转发到http://localhost:81服务;
2.当界面请求接口数据时,只要以/apis 为开头,就会被nginx转发到后端接口服务器上;
总结:nginx实现跨域的原理,实际就是把web项目和后端接口项目放到一个域中,这样就不存在跨域问题,然后根据请求地址去请求不同服务器(真正干活的服务器);
作者:少年仍年少
链接:https://www.jianshu.com/p/8fa2acd103ea
来源:简书
著作权归作者所有。商业转载请联系作者获得授权,非商业转载请注明出处。
#### Overview
The Cross-Origin Resource Sharing standard works by adding new [HTTP headers](https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers) that let servers describe which origins are permitted to read that information from a web browser. Additionally, for HTTP request methods that can cause side-effects on server data (in particular, HTTP methods other than [`GET`](https://developer.mozilla.org/en-US/docs/Web/HTTP/Methods/GET "The HTTP GET method requests a representation of the specified resource. Requests using GET should only retrieve data."), or [`POST`](https://developer.mozilla.org/en-US/docs/Web/HTTP/Methods/POST "The HTTP POST method sends data to the server. The type of the body of the request is indicated by the Content-Type header.") with certain [MIME types](https://developer.mozilla.org/en-US/docs/Web/HTTP/Basics_of_HTTP/MIME_types)), the specification mandates that browsers "preflight" the request, soliciting supported methods from the server with the HTTP [`OPTIONS`](https://developer.mozilla.org/en-US/docs/Web/HTTP/Methods/OPTIONS "The HTTP OPTIONS method is used to describe the communication options for the target resource. The client can specify a URL for the OPTIONS method, or an asterisk (*) to refer to the entire server.") request method, and then, upon "approval" from the server, sending the actual request. Servers can also inform clients whether "credentials" (such as [Cookies](https://developer.mozilla.org/en-US/docs/Web/HTTP/Cookies) and [HTTP Authentication](https://developer.mozilla.org/en-US/docs/Web/HTTP/Authentication)) should be sent with requests.
CORS failures result in errors, but for security reasons, specifics about the error *are not available to JavaScript*. All the code knows is that an error occurred. The only way to determine what specifically went wrong is to look at the browser's console for details.
#### [Server-Side Access Control ](https://developer.mozilla.org/en-US/docs/Web/HTTP/Server-Side_Access_Control)
TP5.1 跨域请求
如果某个路由或者分组需要支持跨域请求,可以使用
~~~php
Route::get('new/:id', 'News/read')
->ext('html')
->allowCrossDomain();
~~~
> 跨域请求一般会发送一条`OPTIONS`的请求,一旦设置了跨域请求的话,不需要自己定义`OPTIONS`请求的路由,系统会自动加上。
跨域请求系统会默认带上一些Header,包括:
~~~php
Access-Control-Allow-Origin:*
Access-Control-Allow-Methods:GET, POST, PATCH, PUT, DELETE
Access-Control-Allow-Headers:Authorization, Content-Type, If-Match, If-Modified-Since, If-None-Match, If-Unmodified-Since, X-Requested-With
~~~
你可以添加或者更改Header信息,使用
~~~php
Route::get('new/:id', 'News/read')
->ext('html')
->header('Access-Control-Allow-Origin','thinkphp.cn')
->header('Access-Control-Allow-Credentials', 'true')
->allowCrossDomain();
~~~
## Method(GET, POST, PUT, DELETE)
### POST
**post使用`multipart/form-data`和`application/x-www-form-urlencoded`的本质区别**
[[参考网页]](https://blog.csdn.net/u013827143/article/details/86222486)
* `application/x-www-form-urlencoded`
1、它是post的默认格式,使用js中`URLencode`转码方法。包括将name、value中的空格替换为加号;将非ascii字符做百分号编码;将input的name、value用‘=’连接,不同的input之间用‘&’连接。
2、百分号编码什么意思呢。比如汉字‘丁’吧,他的utf8编码在十六进制下是0xE4B881,占3个字节,把它转成字符串‘E4B881’,变成了六个字节,每两个字节前加上百分号前缀,得到字符串“%E4%B8%81”,变成九个ascii字符,占九个字节(十六进制下是0x244534254238253831)。把这九个字节拼接到数据包里,这样就可以传输“非ascii字符的 utf8编码的 十六进制表示的 字符串的 百分号形式”,^_^。
3、同样使用URLencode转码,这种post格式跟get的区别在于,get把转换、拼接完的字符串用`?`直接与表单的action连接作为URL使用,所以请求体里没有数据;而post把转换、拼接后的字符串放在了请求体里,不会在浏览器的地址栏显示,因而更安全一些。
* `multipart/form-data`
1、对于一段utf8编码的字节,用`application/x-www-form-urlencoded`传输其中的ascii字符没有问题,但对于非ascii字符传输效率就很低了(汉字‘丁’从三字节变成了九字节),因此在传很长的字节(如文件)时应用`multipart/form-data`格式。`smtp`等协议也使用或借鉴了此格式。
2、此格式表面上发送了什么呢。用此格式发送一段一句话和一个文件,同时请求头里规定了`Content-Type: multipart/form-data; boundary=----WebKitFormBoundarymNhhHqUh0p0gfFa`,请求体里不同的input之间用一段叫boundary的字符串分割,每个input都有了自己一个小header,其后空行接着是数据。
`multipart/form-data`将表单中的每个input转为了一个由boundary分割的小格式,没有转码,直接将utf8字节拼接到请求体中,在本地有多少字节实际就发送多少字节,极大提高了效率,适合传输长字节。
在`Form`元素的语法中,`EncType`表明提交数据的格式。用`Enctype`属性指定将数据回发到服务器时浏览器使用的编码类型。
- `application/x-www-form-urlencoded`: 窗体数据被编码为名称/值对。这是标准的编码格式。
- `multipart/form-data`: 窗体数据被编码为一条消息,页上的每个控件对应消息中的一个部分。
- ` text/plain`: 窗体数据以纯文本形式进行编码,其中不含任何控件或格式字符。
常用的为前两种:`application/x-www-form-urlencoded`和`multipart/form-data`,默认为`application/x-www-form-urlencoded`。
当`action`为`get`时候,浏览器用`x-www-form-urlencoded`的编码方式把form数据转换成一个字串(name1=value1&name2=value2…),然后把这个字串append到url后面,用`?`分割,加载这个新的url。
当`action`为`pos`t时候,浏览器把form数据封装到http body中,然后发送到server。 如果没有`type=file`的控件,用默认的`application/x-www-form-urlencoded`就可以了。 但是如果有`type=file`的话,就要用到`multipart/form-data`了。浏览器会把整个表单以控件为单位分割,并为每个部分加上`Content-Disposition`(form-data或者file),`Content-Type`(默认为text/plain),name(控件name)等信息,并加上分割符(`boundary`).
## 待求证
### 1. Credentials的配置使用
`Access-Control-Allow-Credentials` 头与{domxref(“XMLHttpRequest.withCredentials”)}} 或Fetch API中的Request() 构造器中的`credentials` 选项结合使用。Credentials必须在前后端都被配置(即the `Access-Control-Allow-Credentials` header 和 XHR 或Fetch request中都要配置)才能使带credentials的CORS请求成功。
- WebAPP
- Linux Command
- 入门
- 处理文件
- 查找文件单词
- 环境
- 联网
- Linux
- Linux目录配置标准:FHS
- Linux文件与目录管理
- Linux账号管理与ACL权限设置
- Linux系统资源查看
- 软件包管理
- Bash
- Daemon/Systemd
- ftp
- Apache
- MySQL
- Command
- Replication
- mysqld
- remote access
- remark
- 限制
- PHP
- String
- Array
- Function
- Class
- File
- JAVA
- Protocals
- http
- mqtt
- IDE
- phpDesigner
- eclipse
- vscode
- Notepad++
- WebAPI
- Javasript
- DOM
- BOM
- Event
- Class
- Module
- Ajax
- Fetch
- Promise
- async/await
- Statements and declarations
- Function
- Framwork
- jQurey
- Types
- Promise
- BootStrap
- v4
- ThinkPHP5
- install
- 定时任务
- CodeIgniter
- React.js
- node.js
- npm
- npm-commands
- npm-folder
- package.json
- Docker and private modules
- module
- webpack.js
- install
- configuration
- package.json
- entry
- modules
- plugins
- Code Splitting
- loaders
- libs
- API
- webpack-cli
- Vue.js
- install
- Compile
- VueAPI
- vuex
- vue-router
- vue-devtools
- vue-cli
- vue-loader
- VDOM
- vue-instance
- components
- template
- Single-File Components
- props
- data
- methods
- computed
- watch
- Event-handling
- Render Func
- remark
- 案例学习
- bootstrap-vue
- modal
- fontAwesome
- Hosting Font Awesome Yourself
- using with jquery
- using with Vue.js
- HTML
- CSS
- plugins
- Chart.js
- D3.js
- phpSpreadSheet
- Guzzle
- Cmder
- Git
- git命令
- git流程
- Postman
- Markdown
- Regular Expressions
- PowerDesigner
- 附录1-学习资源