[TOC]
```
npm install sass
编译:$ sass sass/style.scss:css/style.css
监听变化:$ sass --watch sass:css
修改输出格式:
nested -- 嵌套(默认)、compact -- 紧凑、expanded -- 扩展、compressed -- 压缩
$ sass --watch sass:css --style compact
$ sass --watch sass:css --style expanded
$ sass --watch sass:css --style compressed
```
## :-: 3种注解的区别
```
/*
* 多行注释,输出时会保留。
* 注意:如果是压缩将不会被保留
*/
// 单行注释,不会出现在编译之后的css文件内。
/*!
* 强制注释,即便输出形式为压缩。该注释也依然保留、
*/
```
## :-: scss数据类型
:-: 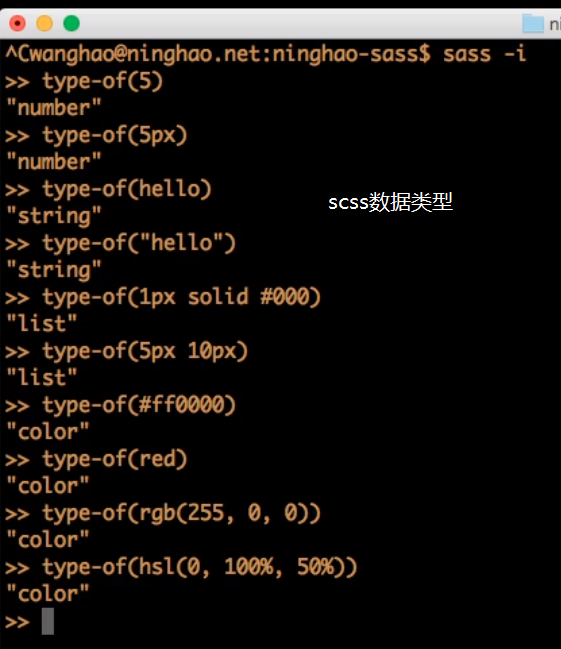
## :-: 定义及使用变量 (数字,颜色,字符串)
```
$primary-color: rgba(0, 0, 0, 0.2);
$primary-border:1px solid $primary-color;
.variable {
background-color: $primary-color;
border: $primary-border;
}
```
## :-: 嵌套语法
```
.box {
.a {
// 嵌套中调用父级 / 引用父级
&:hover {
background: red;
}
&-test-001 {
background: #fff;
}
}
}
// ------------------------------------------- 编译结果
// .box .a:hover { background: red; }
// .box .a-test-001 { background: #fff; }
```
## :-: 属性嵌套
```
.props {
font: {
size: 14px;
weight: 600;
family: 'Courier New', Courier, monospace;
}
// font-size:14px; font-weight:600; ···
border:1px solid #f40 {
left-color: #123;
right-color: #456;
}
// border: 1px solid #f40;
// border-left-color: #123;
// border-right-color: #456;
}
```
## :-: 混合 (类似于js的function)
```
.mixture {
// - 定义
// @mixin 名字(参数1, 参数2...) { ··· }
// - 调用
// @include 名字(#ccc, #fff);
// ··················································
@mixin alert($color) {
// 可以传参、嵌套、引用其他mixin、或者外部变量、
background: $color;
color: #8a6d3b;
a {
color: #bbb;
// darken -- 该方法用于加深颜色;
background: darken($color, 10%);
}
}
.alert-warning {
// 调用
@include alert(#ccc);
@include alert($color: #ccc); // 第二种调用方式,可以无视参数的顺序、
}
}
```
## :-: 继承(扩展)
```
.extend {
.alert {
padding: 5px;
}
.alert a {
color: red; // 也会被继承
}
.alert-info {
@extend .alert; // 继承就是引用一个类的所有样式,作为基础样式。(注意:包括它的子集们)
padding-bottom: 10px;
}
/* .extend .alert, .extend .alert-info { padding: 5px; }
.extend .alert a, .extend .alert-info a { color: red; }
.extend .alert-info { padding-bottom: 10px; } */
}
```
## :-: 模块化
```
.import {
// css3.0 自带导入功能,因为每次@import导入都要发送http请求,这样比较消耗服务器资源。而scss对@import做了优化,将导入的模块都合并在了一个文件。
@import './_base.scss'; // .import a {color: red;}
// partials -- 是将css文件分块,项目一部分。
// 新建一个scss文件(_base.scss),值得注意的是要以下划线'_'开头。这样scss就知道这个是一个partials了。
// 通过@import引入时就不会编译成两份文件而是合并在一起了。
}
```
## :-: 数据类型
### :-: 数字
```
.Numbers {
// 基础运算
width: 1 / 3 * 100%; // 33.3333333333%
width: (200 / 4) + px; // 50px
height: 5 * 5 + px; // 25px
// 通过数字函数
// abs -- 绝对值
padding: abs(-10px); // 10px
// round -- 四舍五入
padding: round(3.5px); // 4px
// ceil -- 向上取整
padding: ceil(3.1px); // 4px
// floor -- 向下取整
padding: floor(3.9px); // 3px
// percentage -- 取百分比
padding: percentage(65px / 100px); // 65%
margin: min(1, 2, 3); // 测试了没效果 - 1
margin: max(1, 2, 3); // 测试了没效果 - 3
}
```
### :-: 字符串
```
.string {
// 输出的形式不同
width: 'abc' + def; // "abcdef"
width: abc + 'def'; // abcdef
width: abc + def; // abcdef
width: 1+'px'; // "1px"
width: 1+px; // 1px
// 字符串函数
$greeting: 'Hello World~';
// to-upper-case -- 转大写
top: to-upper-case($greeting); // "HELLO WORLD~"
// to-lower-case -- 转小写
top: to-lower-case($greeting); // "hello world~"
// str-length -- 返回字符串的长度
top: str-length($greeting); // 12
// str-index -- 返回指定片段的索引位,起点为1。注意:如果没有不会输出。
top: str-index($greeting, "World"); // 7
// str-insert -- 插入字符片段,索引从1开始。可以为负数。-1 为最后一个。
top: str-insert($greeting, '.net', -1); // Hello World~.net
}
```
### :-: 颜色
```
.color {
// rgb(红, 绿, 蓝) 范围:0 ~ 255
// rgba(红, 绿, 蓝, 不透明度) 范围:0 ~ 255 、不透明度:0 ~ 1.0
color: rgb(255, 0, 0); // red
// 在scss中rgb可以直接用百分比表示
color: rgba(80%, 0, 0, 30%); // #cc0000
// hsl 色相(0deg~360deg)、饱和度(0~100%)、明度(0~100%)
// hsla 色相(0deg~360deg)、饱和度(0%~100%)、明度(0%~100%)、不透明度(0~1.0)
color: hsl(0, 100%, 50%); // red
// 颜色函数
$color: #f00;
$color-hsl: hsl(0, 100%, 50%);
// adjust-hue -- 用于调整色相
color: adjust-hue($color, 45deg); // #ffbf00
// lighten -- 增加颜色明度。
color: lighten($color, 20%); // #ff6666
// darken -- 减少颜色明度。
color: darken($color, 20%); // #990000
// 纯度(饱和度)
// saturate -- 提高纯度
color: saturate($color, 20%);
// desaturate -- 降低纯度
color: desaturate($color, 20%);
// 不透明度
// opacify -- 增加颜色不透明度
color: opacify($color, 0.3);
// opacify -- 减少颜色不透明度
color: transparentize($color, 0.3);
}
```
### :-: 列表
```
.list {
// 分隔符 ',' | ' ' | '()'
$list: 5px 10px 0 5px;
// length -- 返回列表的长度
top: length($list); // 4
// nth -- 获取指定索引位的值
top: nth($list, 2); // 10px
// index -- 匹配该索引在列表中的索引位
top: index($list, 5px); // 1
// append -- 向后插入成员,相当于js的arr.push
top: append($list, 1px); // 5px 10px 0 5px 1px
// join -- 合并列表,第三个参数可以指定分隔符。
content: join($list, 6px 7px, comma); // 5px, 10px, 0, 5px, 6px, 7px
// map 命名列表
$list: (key: #f40, dark: #000);
// map-get -- map取值
color: map-get($list, dark); // #000
// map-keys -- 获取map所有的属性名
color: map-keys($list); // key, dark
// map-values -- 获取map所有的值
color: map-values($list); // #f40, #000
// map-has-key -- 判断map中是否有指定的key。返回 true/false
color: map-has-key($list, key); // true
// map-merge -- 合并两个map为一个、
$list: map-merge($list, (a: 1px, b: 2px));
color: map-get($list, b); // 2px
// map-remove -- map移除指定成员,支持移除多个
$list: map-remove($list, a, b, dark);
}
```
### :-: 布尔值
```
.Boolean {
top: 12px > 2px;
// not -- 取反
top: not(true);
top: (1px)>(2px) and (2px)<(1px);
top: (1px)>(2px) or (2px)<(1px);
}
```
## :-: 模版字符串(Interpolation)
```
$version:1.0.1.20180927;
/* 当前版本号:#{$version} */ // 当前版本号:1 0.1 0.20180927
.test-::before {
content: "#{$version}"; // "1 0.1 0.20180927"
}
$str:"A001";
.test-#{$str} {top: 0;} // .test-A001
```
## :-: 流程控制
### :-: if
```
// @if 条件 {···}
.if {
// - @if -- 当结果为true时,才会被包含
// - @else if - @else
$index: 1;
@if $index==0 { color: red; }
@else if $index==1 { color: #000; }
@else { color: #fff; }
}
```
### :-: for
```
// @for $var from <开始值> through <结束值> { ··· }
// @for $var from <开始值> to <结束值> { ··· }
.for {
// through 跟 to 的区别,to 不会执行最后一次。如结束值为5,to - 5 | through - 4
$len: 3;
@for $i from 1 through $len {
.col-#{$i} { width: 100% / $len * $i; }
}
@for $i from 1 to $len { index: $i + px; }
}
```
### :-: each
```
// @each $var in $list { ··· }
.each {
// @each -- 遍历列表
$list: a b c d e f;
@each $v in $list {
.icon {
background-image: url('./image/#{$v}.png');
}
}
}
```
### :-: while
```
// @while 条件 { ··· }
.while {
$i: 1;
@while $i<=5 {
top: $i + px;
$i: $i + 1;
}
}
```
### :-: function / alert
```
// @function 名字 (参数1,参数2) { ··· }
.function {
// 用户自定义函数
@function color () { @return 123px; }
padding-top: color(); // 123px
// 提示信息
@function alert () {
@warn 'Warning'; // 在命令行中提示
@error 'Error'; // 终止解析,抛出错误
@return 1px;
}
top: alert();
}
```
## :-: 循环
```
@for $i from 0 through 24 {
.el-col-#{$i} {
width: $i / 24 * 100%;
}
}
```
- 前端工具库
- HTML
- CSS
- 实用样式
- JavaScript
- 模拟运动
- 深入数组扩展
- JavaScript_补充
- jQuery
- 自定义插件
- 网络 · 后端请求
- css3.0 - 2019-2-28
- 选择器
- 边界样式
- text 字体系列
- 盒子模型
- 动图效果
- 其他
- less - 用法
- scss - 用法 2019-9-26
- HTML5 - 2019-3-21
- canvas - 画布
- SVG - 矢量图
- 多媒体类
- H5 - 其他
- webpack - 自动化构建
- webpack - 起步
- webpack -- 环境配置
- gulp
- ES6 - 2019-4-21
- HTML5补充 - 2019-6-30
- 微信小程序 2019-7-8
- 全局配置
- 页面配置
- 组件生命周期
- 自定义组件 - 2019-7-14
- Git 基本操作 - 2019-7-16
- vue框架 - 2019-7-17
- 基本使用 - 2019-7-18
- 自定义功能 - 2019-7-20
- 自定义组件 - 2019-7-22
- 脚手架的使用 - 2019-7-25
- vue - 终端常用命令
- Vue Router - 路由 (基础)
- Vue Router - 路由 (高级)
- 路由插件配置 - 2019-7-29
- 路由 - 一个实例
- VUEX_数据仓库 - 2019-8-2
- Vue CLI 项目配置 - 2019-8-5
- 单元测试 - 2019-8-6
- 挂载全局组件 - 2019-11-14
- React框架
- React基本使用
- React - 组件化 2019-8-25
- React - 组件间交互 2019-8-26
- React - setState 2019-11-19
- React - slot 2019-11-19
- React - 生命周期 2019-8-26
- props属性校验 2019-11-26
- React - 路由 2019-8-28
- React - ref 2019-11-26
- React - Context 2019-11-27
- PureComponent - 性能优化 2019-11-27
- Render Props VS HOC 2019-11-27
- Portals - 插槽 2019-11-28
- React - Event 2019-11-29
- React - 渲染原理 2019-11-29
- Node.js
- 模块收纳
- dome
- nodejs - tsconfig.json
- TypeScript - 2020-3-5
- TypeScript - 基础 2020-3-6
- TypeScript - 进阶 2020-3-9
- Ordinary小助手
- uni-app
- 高德地图api
- mysql
- EVENTS
- 笔记