[TOC]
## :-: [React官网](https://www.reactjscn.com/)
*****
## :-: 准备工作
### 使用脚手架搭建工程
- 官方:create-react-app
- 第三方:next.js、umijs
### React工具及插件
- React开发者插件(浏览器) -- React Developer Tools
- 代码风格检查 -- ESLint
- ES7代码快 -- ES7 React/Redux/GraphQL/React-Native snippets
### 让js支持html代码块 (jsx)
```
// VSCode 设置中配置 emmet
"emmet.includeLanguages": {
"wxml": "html",
"javascript":"javascriptreact"
},
```
## :-: 基本命令
```
-- 全局安装React脚手架
$ npm install create-react-app -g
-- 创建React项目'test-name'为项目名。
$ create-react-app test-name
React项目下的命令
- 开启一个服务
$ npm start
- 编译React项目,上线打包
$ npm run build
- 开启测试环境
$ npm test
- 移除所有的工具(谨慎使用)
$ npm run eject
-- 打开VSCode编辑器
$ code .
```
:-: jsx语法 == js + xml(html)
## :-: Demo
:-: index.js
```
import React from "react";
import { render } from "react-dom";
import { topList } from "./data.js";
// 引入css
import "./index.css";
function test() {
return "Hello World~";
}
// <React.Fragment></React.Fragment> -- 包裹标签 或者可以这样写'<>内容区</>'
// 在jsx中、 { js } < html >
// 关键字处理:class -- className 、 for -- htmlFor
// class是关键字,需要写成className
// for也是关键字,需要写成htmlFor。<label for="inp"></label><input id="inp" type="text"/>
const html = (
<div style={{ width: "230px", backgroundColor: "#666", marginTop: "10px" }}>
{test()}
{JSON.stringify({ a: 123, b: "456", c: true })}
{[<p key="0">---------------------</p>, 1, 2, 3]}
</div>
),
label = (
<>
<label htmlFor="inp">激活</label>
<input id="inp" type="text" />
</>
);
let element = (
<React.Fragment>
<div
style={{
margin: "20px",
border: "1px solid red",
padding: "20px",
width: "230px"
}}
>
{label}
{html}
</div>
<div className="wrapper">
<div className="search-title-box">
<h5 className="search-title">搜索热点</h5>
<span className="refresh">换一换</span>
</div>
<ul className="top-list-container">
{topList.map((item, index) => {
const indexStyle = {};
switch (index) {
case 0:
indexStyle.backgroundColor = "#f54545";
break;
case 1:
indexStyle.backgroundColor = "#ff8547";
break;
case 2:
indexStyle.backgroundColor = "#ffac38";
break;
default:
break;
}
return (
<li className="top-list" key={item.id}>
<div className="top-title">
<span className="hot-index" style={indexStyle}>
{index + 1}
</span>
<a href="/" className="topic-title">
{item.title}
</a>
{item.new && <span className="topic-new">新</span>}
</div>
<div className="hot-degree">
<span>{item.hot}</span>
</div>
</li>
);
})}
</ul>
</div>
</React.Fragment>
);
// 渲染标签、innerHTML、相当于vue中的 v-html (危险的 设置 HTML)
let str = "<span>我是HTML</span>";
let testDom = <div dangerouslySetInnerHTML={{ __html: str }} />;
render(element, document.getElementById("root"));
```
:-: data.js
```
const topList = [
{ id: 0, title: "老年人才用9键", new: true, hot: "46万" },
{ id: 1, title: "人贩子张维平死刑", new: true, hot: "44万" },
{ id: 2, title: "全国冻哭预警地图", new: false, hot: "35万" },
{ id: 3, title: "沈梦辰晒婚纱照", new: false, hot: "33万" },
{ id: 4, title: "恋爱4个月胖50近", new: true, hot: "32万" },
{ id: 5, title: "郭麒麟初中早恋", new: false, hot: "25万" },
{ id: 6, title: "男孩滑雪遭遇雪崩", new: true, hot: "24万" },
{ id: 7, title: "大熊猫玩菜刀", new: false, hot: "24万" },
{ id: 8, title: "姿态宣布退役", new: false, hot: "22万" },
{ id: 9, title: "卫龙辣条吃出虫子", new: false, hot: "20万" },
{ id: 10, title: "女生被罚抱头蹲", new: true, hot: "20万" }
];
export { topList };
```
:-: 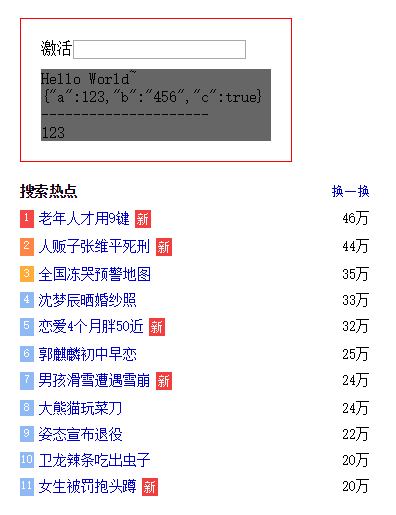
- 前端工具库
- HTML
- CSS
- 实用样式
- JavaScript
- 模拟运动
- 深入数组扩展
- JavaScript_补充
- jQuery
- 自定义插件
- 网络 · 后端请求
- css3.0 - 2019-2-28
- 选择器
- 边界样式
- text 字体系列
- 盒子模型
- 动图效果
- 其他
- less - 用法
- scss - 用法 2019-9-26
- HTML5 - 2019-3-21
- canvas - 画布
- SVG - 矢量图
- 多媒体类
- H5 - 其他
- webpack - 自动化构建
- webpack - 起步
- webpack -- 环境配置
- gulp
- ES6 - 2019-4-21
- HTML5补充 - 2019-6-30
- 微信小程序 2019-7-8
- 全局配置
- 页面配置
- 组件生命周期
- 自定义组件 - 2019-7-14
- Git 基本操作 - 2019-7-16
- vue框架 - 2019-7-17
- 基本使用 - 2019-7-18
- 自定义功能 - 2019-7-20
- 自定义组件 - 2019-7-22
- 脚手架的使用 - 2019-7-25
- vue - 终端常用命令
- Vue Router - 路由 (基础)
- Vue Router - 路由 (高级)
- 路由插件配置 - 2019-7-29
- 路由 - 一个实例
- VUEX_数据仓库 - 2019-8-2
- Vue CLI 项目配置 - 2019-8-5
- 单元测试 - 2019-8-6
- 挂载全局组件 - 2019-11-14
- React框架
- React基本使用
- React - 组件化 2019-8-25
- React - 组件间交互 2019-8-26
- React - setState 2019-11-19
- React - slot 2019-11-19
- React - 生命周期 2019-8-26
- props属性校验 2019-11-26
- React - 路由 2019-8-28
- React - ref 2019-11-26
- React - Context 2019-11-27
- PureComponent - 性能优化 2019-11-27
- Render Props VS HOC 2019-11-27
- Portals - 插槽 2019-11-28
- React - Event 2019-11-29
- React - 渲染原理 2019-11-29
- Node.js
- 模块收纳
- dome
- nodejs - tsconfig.json
- TypeScript - 2020-3-5
- TypeScript - 基础 2020-3-6
- TypeScript - 进阶 2020-3-9
- Ordinary小助手
- uni-app
- 高德地图api
- mysql
- EVENTS
- 笔记