## String Character Functions
The [String](https://www.arduino.cc/en/Reference/StringObject) functions `charAt()` and `setCharAt()` are used to get or set the value of a character at a given position in a String.
At their simplest, these functions help you search and replace a given character. For example, the following replaces the colon in a given String with an equals sign:
String reportString = "SensorReading: 456";
int colonPosition = reportString.indexOf(':');
reportString.setCharAt(colonPosition, '=');
Here's an example that checks to see if the first letter of the second word is 'B':
String reportString = "Franklin, Benjamin";
int spacePosition = reportString.indexOf(' ');
if (reportString.charAt(spacePosition + 1) == 'B') {
Serial.println("You might have found the Benjamins.")
}
Caution: If you try to get the `charAt` or try to `setCharAt()` a value that's longer than the String's length, you'll get unexpected results. If you're not sure, check to see that the position you want to set or get is less than the string's length using the `length()` function.
### Hardware Required
* Arduino or Genuino Board
### Circuit
There is no circuit for this example, though your board must be connected to your computer via USB and the serial monitor window of the Arduino Software (IDE) should be open.
[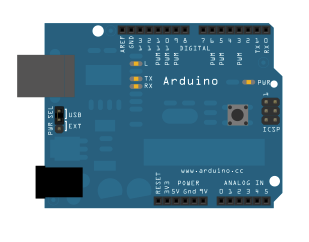](https://www.arduino.cc/en/uploads/Tutorial/Arduino_bb.png)
image developed using [Fritzing](http://www.fritzing.org/). For more circuit examples, see the [Fritzing project page](http://fritzing.org/projects/)
### Code
/*
String charAt() and setCharAt()
Examples of how to get and set characters of a String
created 27 Jul 2010
modified 2 Apr 2012
by Tom Igoe
This example code is in the public domain.
http://www.arduino.cc/en/Tutorial/StringCharacters
*/
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
Serial.println("\n\nString charAt() and setCharAt():");
}
void loop() {
// make a String to report a sensor reading:
String reportString = "SensorReading: 456";
Serial.println(reportString);
// the reading's most significant digit is at position 15 in the reportString:
char mostSignificantDigit = reportString.charAt(15);
String message = "Most significant digit of the sensor reading is: ";
Serial.println(message + mostSignificantDigit);
// add blank space:
Serial.println();
// you can also set the character of a String. Change the : to a = character
reportString.setCharAt(13, '=');
Serial.println(reportString);
// do nothing while true:
while (true);
}
[[Get Code]](https://www.arduino.cc/en/Tutorial/StringCharacters?action=sourceblock&num=1)
### See Also
* [String object](https://www.arduino.cc/en/Reference/StringObject) – Your Reference for String objects
* [CharacterAnalysis](https://www.arduino.cc/en/Tutorial/CharacterAnalysis) - We use the operators that allow us to recognise the type of character we are dealing with.
* [StringAdditionOperator](https://www.arduino.cc/en/Tutorial/StringAdditionOperator) - Add strings together in a variety of ways.
* [StringAppendOperator](https://www.arduino.cc/en/Tutorial/StringAppendOperator) - Use the += operator and the concat() method to append things to Strings
* [StringCaseChanges](https://www.arduino.cc/en/Tutorial/StringCaseChanges) - Change the case of a string.
* [StringComparisonOperators](https://www.arduino.cc/en/Tutorial/StringComparisonOperators) - Get/set the value of a specific character in a string.
* [StringConstructors](https://www.arduino.cc/en/Tutorial/StringConstructors) - Initialize string objects.
* [StringIndexOf](https://www.arduino.cc/en/Tutorial/StringIndexOf) - Look for the first/last instance of a character in a string.
* [StringLength](https://www.arduino.cc/en/Tutorial/StringLength) - Get the length of a string.
* [StringLengthTrim](https://www.arduino.cc/en/Tutorial/StringLengthTrim) - Get and trim the length of a string.
* [StringReplace](https://www.arduino.cc/en/Tutorial/StringReplace) - Replace individual characters in a string.
* [StringStartsWithEndsWith](https://www.arduino.cc/en/Tutorial/StringStartsWithEndsWith) - Check which characters/substrings a given string starts or ends with.
* [StringSubstring](https://www.arduino.cc/en/Tutorial/StringSubstring) - Look for "phrases" within a given string.
* [StringToInt](https://www.arduino.cc/en/Tutorial/StringToInt) - Allows you to convert a String to an integer number.
- 说明
- 系统示例文件目录结构及说明
- 01.Basics
- AnalogReadSerial
- BareMinimum
- Blink
- DigitalReadSerial
- Fade
- ReadAnalogVoltage
- 02.Digital
- BlinkWithoutDelay
- Button
- Debounce
- DigitalInputPullup
- StateChangeDetection
- toneKeyboard
- toneMelody
- toneMultiple
- tonePitchFollower
- 03.Analog
- AnalogInOutSerial
- AnalogInput
- AnalogWriteMega
- Calibration
- Fading
- Smoothing
- 04.Communication
- ASCIITable
- Dimmer
- Graph
- Midi
- MultiSerial
- PhysicalPixel
- ReadASCIIString
- SerialCallResponse
- SerialCallResponseASCII
- SerialEvent
- SerialPassthrough
- VirtualColorMixer
- 05.Control
- Arrays
- ForLoopIteration
- IfStatementConditional
- switchCase
- switchCase2
- WhileStatementConditional
- 06.Sensors
- ADXL3xx
- Knock
- Memsic2125
- Ping
- 07.Display
- barGraph
- RowColumnScanning
- 08.Strings
- CharacterAnalysis
- StringAdditionOperator
- StringAppendOperator
- StringCaseChanges
- StringCharacters
- StringComparisonOperators
- StringConstructors
- StringIndexOf
- StringLength
- StringLengthTrim
- StringReplace
- StringStartsWithEndsWith
- StringSubstring
- StringToInt
- 09.USB
- Keyboard
- KeyboardLogout
- KeyboardMessage
- KeyboardReprogram
- KeyboardSerial
- KeyboardAndMouseControl
- Mouse
- ButtonMouseControl
- JoystickMouseControl
- 10.StarterKit_BasicKit (与特定硬件相关,暂无)
- p02_SpaceshipInterface
- p03_LoveOMeter
- p04_ColorMixingLamp
- p05_ServoMoodIndicator
- p06_LightTheremin
- p07_Keyboard
- p08_DigitalHourglass
- p09_MotorizedPinwheel
- p10_Zoetrope
- p11_CrystalBall
- p12_KnockLock
- p13_TouchSensorLamp
- p14_TweakTheArduinoLogo
- p15_HackingButtons
- 11.ArduinoISP(暂无)
- ArduinoISP