国际化就是根据需要能够将网站的语言切换为当地语言信息,如可以将网站在中英文之间进行切换。
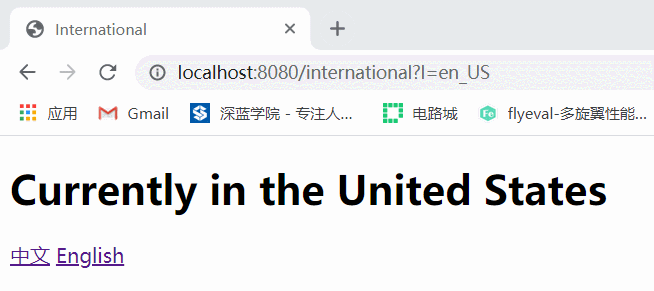
我们可以实现根据浏览器使用的默认语言来实现网站语言的切换,或定制我们的区域信息解析器来实现点击链接切换语言。
<br/>
步骤如下:
**1. 明确需要国际化的地方**
假如我需要将`templates/international.html`页面进行国际化。
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<!-- international.title 是下面国际化配置文件的属性名 -->
<title th:text="#{international.title}">国际化</title>
</head>
<body>
<h1 th:text="#{international.location}">当前在中国</h1>
<!-- 需要传递一个l的参数让后台知道要使用的是哪种语言 -->
<a th:href="@{/international(l=zh_CN)}">中文</a>
<a th:href="@{/international(l=en_US)}">English</a>
</body>
</html>
```
**2. 编写国际化配置文件**
国际化配置文件统一使用`.properties`来编写,命令规则为:`页面名_语言_国家编码`,如中文配置文件:`international_zh_CN.properties`、英文(美国)`international_en_US.properties`。
先创建三个空的国际化配置文件,如下图:
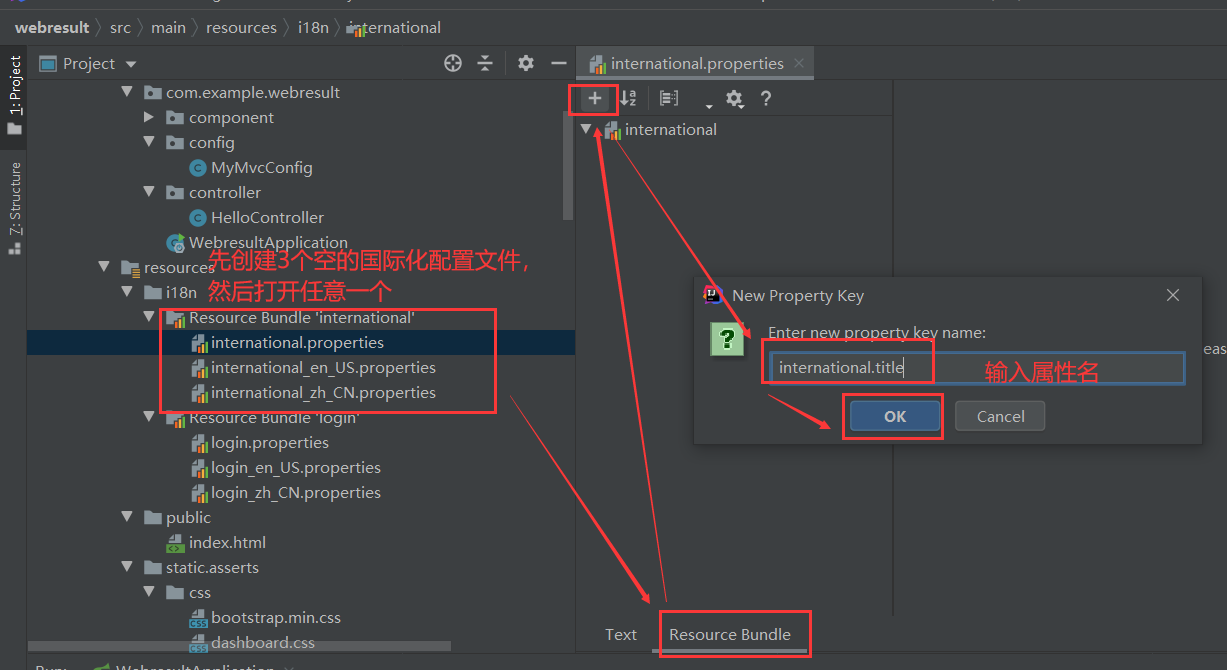
`international.properties`的作用是当没有提供对应的国际化配置文件时,默认采用该配置文件。
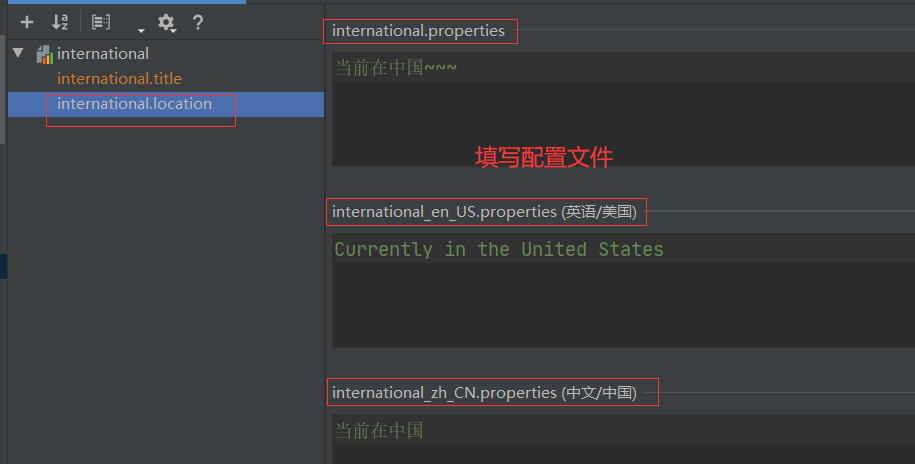
最终填写如下:
**`resources/i18n/international.properties`**
```xml
international.location=当前在中国~~~
international.title=国际化~~~~
```
**`resources/i18n/international_zh_CN.properties`**
```xml
international.location=当前在中国
international.title=国际化
```
**`resources/i18n/international_en_US.properties`**
```xml
international.location=Currently in the United States
international.title=International
```
**3. 指定国际化配置文件的位置**
国际化配置文件默认在`resources`目录,但是我们更改为`resources/i18n/`目录,所以需要指定。
**`resources/application.properties`**
```xml
# 当你有多个国际化配置文件时用 , 隔开
spring.messages.basename=i18n.login, i18n.international
```
**4. 启动项目访问`international.html`页面**
当你访问到该页面,由浏览器的默认语言来决定使用哪个配置文件。下图为我的谷歌浏览器默认的语言为简体中文,所以采用是`international_zh_CN.properties`的配置信息。
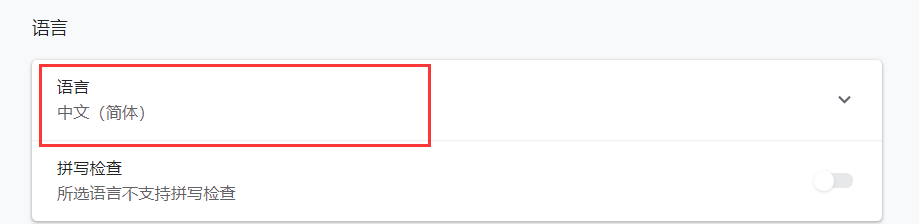
上面网站的语言由浏览器当前的默认语言决定,但是我们可以在页面放置链接任意在中英文之间切换。
其原理是:可以使用区域信息解析器LocaleResolver来获取国际化Locale,它的部分源码如下:
```java
-----WebMvcAutoConfiguration-----
public LocaleResolver localeResolver() {
// 如果没有指定区域信息,则使用默认的FIXED
if (this.mvcProperties.getLocaleResolver() ==
org.springframework.boot.autoconfigure.web.servlet.WebMvcProperties.LocaleResolver.FIXED) {
return new FixedLocaleResolver(this.mvcProperties.getLocale());
} else {
// 如果没有指定,则从AcceptHeaderLocaleResolver获取区域信息
AcceptHeaderLocaleResolver localeResolver = ▲▲▲▲new AcceptHeaderLocaleResolver();▲▲▲▲
localeResolver.setDefaultLocale(this.mvcProperties.getLocale());
return localeResolver;
}
}
-----AcceptHeaderLocaleResolver-----
public Locale resolveLocale(HttpServletRequest request) {
Locale defaultLocale = this.getDefaultLocale();
if (defaultLocale != null && request.getHeader("Accept-Language") == null) {
return defaultLocale;
} else {
// 从请求头中获取区域信息
▲▲▲▲Locale requestLocale = request.getLocale();▲▲▲▲
List<Locale> supportedLocales = this.getSupportedLocales();
if (!supportedLocales.isEmpty() && !supportedLocales.contains(requestLocale)) {
Locale supportedLocale = this.findSupportedLocale(request, supportedLocales);
if (supportedLocale != null) {
return supportedLocale;
} else {
return defaultLocale != null ? defaultLocale : requestLocale;
}
} else {
return requestLocale;
}
}
}
```
在浏览器中按F12,找到任意一个请求,可以看到请求头部的语言信息,我们需要定制自己的信息解析器来解析的请求头部信息。
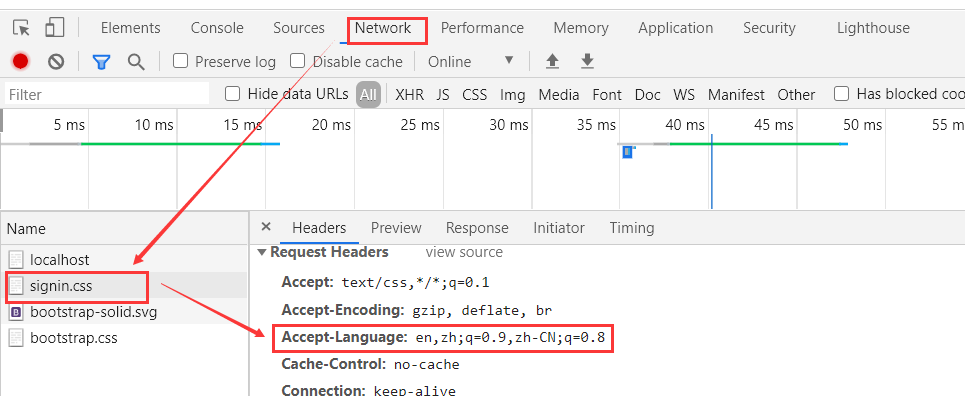
**5. 实现我们自己的区域信息解析器**
*`com.example.webresult.component.MyLocaleResolver`*
```java
package com.example.webresult.component;
import org.apache.tomcat.jni.Local;
import org.springframework.web.servlet.LocaleResolver;
import org.thymeleaf.util.StringUtils;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.Locale;
public class MyLocaleResolver implements LocaleResolver {
@Override
public Locale resolveLocale(HttpServletRequest request) {
// 获取从前端请求的参数l
String l = request.getParameter("l");
// 如果没有请求参数l,则使用默认区域
Locale local = Locale.getDefault();
if (!StringUtils.isEmpty(l)) {
String[] split = l.split("_");
// 创建我们自己的区域,(国家语言, 国家编码)
local = new Locale(split[0], split[1]);
}
return local;
}
@Override
public void setLocale(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Locale locale) {
}
}
```
**6. 将定制的区域信息解析器注册到IoC容器中**
需要将上面定制的区域信息解析器注册到IoC容器中,让我们的区域信息解析器起效,覆盖SpringMVC默认的解析器。
*`com.example.webresult.config.MyMvcConfig`*
```java
package com.example.webresult.config;
import com.example.webresult.component.MyLocaleResolver;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.LocaleResolver;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
// 如果使用@EnableWebMvc则是完全取代该父类的自动配置
// @EnableWebMvc
@Configuration
public class MyMvcConfig implements WebMvcConfigurer {
/**
* 将我们的区域解析器注入到IoC容器中,覆盖SpringMVC默认的解析器
* @return
*/
@Bean
public LocaleResolver localeResolver() {
return new MyLocaleResolver();
}
}
```
**7. 我编写的controller如下**
*`com.example.webresult.controller.IndexController`*
```java
package com.example.webresult.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class HelloController {
@RequestMapping(value = "/international")
public String international() {
return "international";
}
}
```
启动项目后访问:http://localhost:8080/international 显示如下:
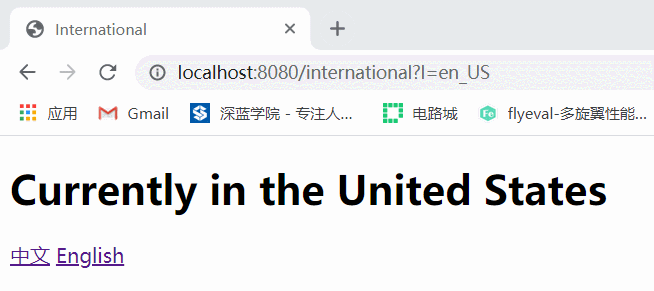
- Mybatis
- mybatis是什么
- mybatis优缺点
- 环境搭建
- 使用步骤
- 传参方式
- 无需传参
- 一个参数
- 多个参数
- 增/删/改
- 查询
- 单表查询
- 一对一查询
- 一对多查询
- 动态SQL
- 注解操作
- Spring
- Spring什么
- Spring优点
- Spring组成
- 第一个Spring程序
- 两大核心技术
- IoC控制反转
- IoC思想
- IoC容器使用步骤
- 属性注入
- IoC注入方式
- 模拟IoC实现
- AOP
- AOP概念
- AOP原理
- AOP关键术语
- AOP编程过程
- 切入点规则
- 5种增强方式
- Spring注解开发
- 注解开发的优势
- Bean注解开发
- AOP注解开发
- 完全注解开发
- 模拟Spring注解开发
- 自动装配
- 配置文件拆分
- SpringBean
- Bean常用属性
- Bean的作用域
- Bean的生命周期
- Spring整合MyBatis
- 整合步骤
- SqlSessionTemplate
- 业务层添加事务
- 事务的作用
- 配置文件事务
- 注解事务
- 事务参数
- SpringMVC
- SpringMVC是什么
- 环境搭建
- 请求流程
- 核心组件
- 前后端交互
- 简单交互演示
- 常用注解
- 后端数据传递至前端
- ServletAPI
- 访问静态资源
- 异常处理
- HandlerExceptionResolver
- 局部异常
- 全局异常
- 转发与重定向
- 转发演示
- 重定向演示
- 转发与重定向的区别
- 获取表单数据
- 表单标签
- REST风格的URL
- 异步处理
- 异步请求
- JSON数据处理
- 中文乱码处理
- 日期处理
- 上传文件
- 拦截器
- 视图解析器
- 视图类型
- 多视图解析器
- 自定义pdf视图
- JSR303数据验证
- JSR303是什么
- 常用约束
- 使用步骤
- SpringMVC整合Mybatis
- 整合步骤
- Mybatis分页插件
- SpringBoot
- SpringBoot是什么
- 环境搭建
- SpringBoot启动分析
- SpringBoot启动类
- 启动过程
- SpringBoot配置文件
- 配置文件类型
- 更改配置文件
- 读取配置文件
- 占位符
- 配置优先级
- 自定义IoC容器
- 定义方式
- 引入Spring配置文件
- @Configuration
- SpringBoot自动配置
- 自动配置原理
- 条件注解
- 自动配置报告
- 自定义自动配置
- 关闭自动配置
- 接管自动配置
- 多环境配置
- CommandLineRunner
- SpringBoot与Web开发
- 引入模板引擎
- Thymeleaf模板
- Freemarker模板
- 静态资源访问
- webjars
- 静态资源位置
- ico图标
- 指定首页
- 更换Web服务器
- 国际化
- 拦截器
- 错误处理机制
- 错误处理机制原理
- 定制错误页面
- 定制错误数据
- 上传文件
- 注册servlet三大组件
- 注册Servlet
- 注册过滤器
- 注册监听器
- 外部Tomcat与jsp模板
- 前后端交互
- 传递json字符串
- 传递js对象
- 传递表单
- 下载功能
- Swagger2文档
- SpringBoot整合JDBC
- 整合步骤
- 核心API
- JdbcTemplate
- 增删改
- 查询
- NamedParameterJdbcTemplate
- 增删改
- 查询
- SpringBoot整合Mybatis
- 整合步骤
- 切换为Druid数据源
- 添加事务
- Mybatis分页插件
- 场景启动器
- 场景启动器是什么
- 自定义场景启动器
- SpringBoot与日志
- 日志框架
- slf4j日志
- slf4j日志实现
- 统一切换为slf4j
- 日志配置
- 日志文件
- 切换日志框架
- 切换日志场景启动器
- SpringBoot与缓存
- JSR107缓存技术
- Spring缓存抽象
- 缓存注解
- SpEL表达式
- 使用缓存
- 自定义key生成器
- 缓存工作原理与流程
- SpringBoot整合Redis
- 整合步骤
- 初步使用
- 序列化机制
- 缓存管理器
- SpringBoot与任务
- 异步任务
- 实现异步任务
- 注意事项与原理
- 自定义线程池
- 定时任务
- cron表达式
- 创建定时任务
- @Scheduled参数
- 动态时间
- 邮件任务
- Quartz定时任务
- Quartz是什么
- 创建定时任务
- 触发器与任务
- 任务的CURD
- 两种触发器
- 并发问题
- 持久化
- 任务持久化
- Quartz集群
- misfire策略
- 打包插件
- appassembler-maven-plugin
- appassembler与assembly配合