## JS基础课阶段复习
### 一、基础知识问答
#### 1.
JS中的数据类型包括:
* 基本数据类型
* number
* string
* boolean
* null
* undefined
* symbol
* bigint
* 引用数据类型
* object
* {} 普通对象
* [] 数组对象
* /^$/ 正则对象
* Math
* 日期对象
* …
* function
> 基本数据类型是按照值操作,直接把值存储到栈内存中即可;引用数据类型的值是存储到堆内存中的,我们操作的都是堆内存的引用地址;
#### 2.
JS中检测数据类型:
* typeof
* instanceof
* constructor
* Object.prototype.toString.call()
#### 3.
浏览器常用的内核:
* webkit
* gecko
* trident
* blink
#### 4.
JS中创建变量的几种常用方式:
* var
* let / const
* function
* class
* import
#### 5.
null和undefined的区别:
#### 6.
~~~
let n = {
name:'珠峰培训'
};
let m = n;
n.teacher = n = {
name:'周啸天'
};
m.teacher = {
name:'耿银鹏'
};
console.log(m.name); //“珠峰培训”
console.log(n.name); // “周啸天”
console.log(m.teacher.name); // “耿银鹏”
console.log(n.teacher.name); // 报错 Uncaught TypeError: Cannot read property 'name' of undefined
~~~
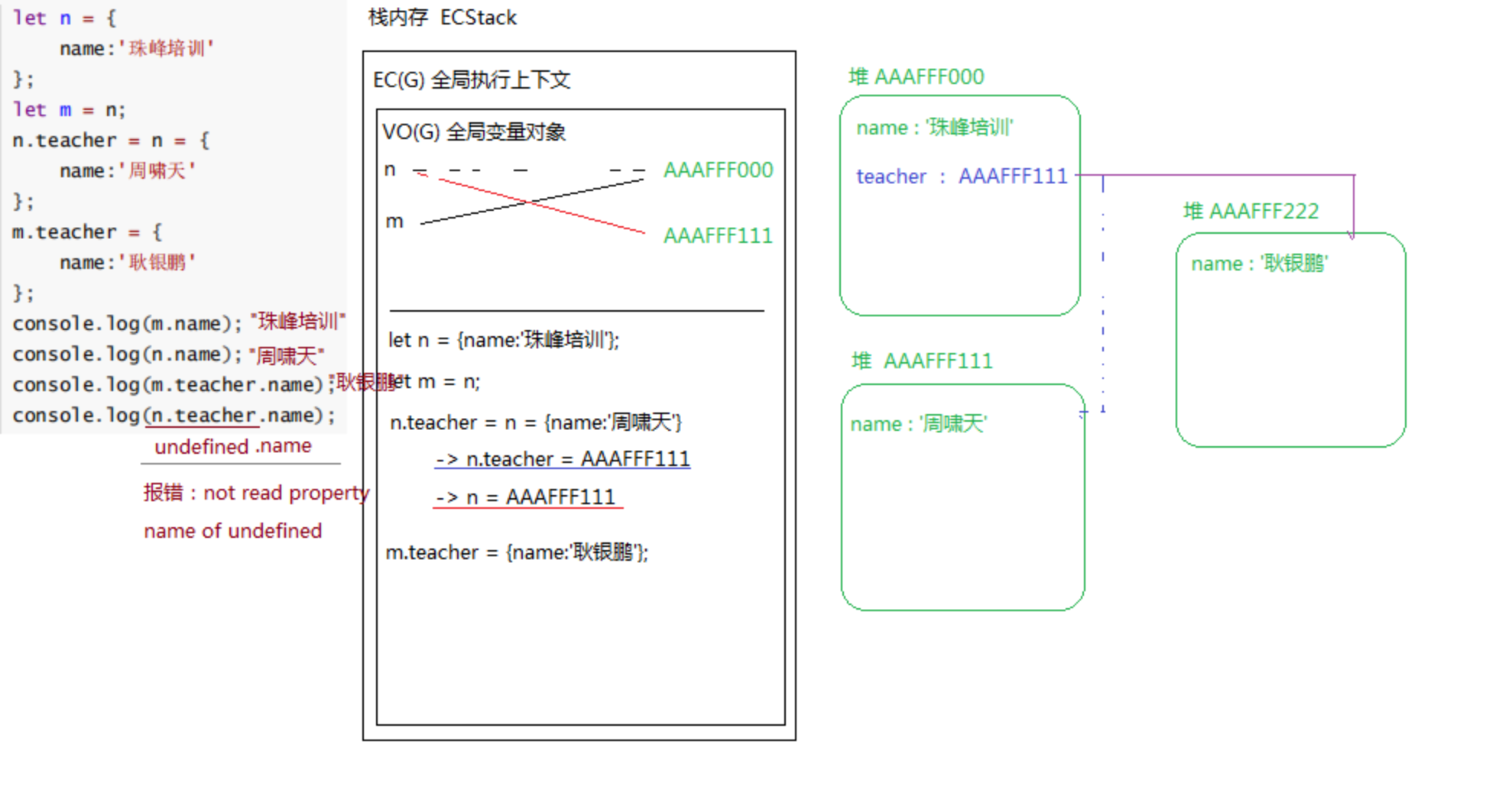
#### 7.
‘number’
~~~javascript
let num = Number('12.5px'); //=>NaN
let type = typeof num; //=>"number"
if (num == NaN) { alert(NaN); }
else if (type === 'number') { alert('number'); }
else if (num == 12.5) { alert(12.5); }
else { alert('12.5px'); }
~~~
#### 8.
```
console.log(1 + false + undefined + [] + null + true + '珠峰培训' + [12]+ 1);
let i=1;
console.log(2-(i++)+(--i)+2+(++i)-(i--)-2);
console.log(i);
```
“NaNnulltrue珠峰培训121”
2 1
~~~javascript
//=>加号在JS中除了数学运算还有字符串拼接(加号左右两边出现字符串或者对象,基本上都是字符串拼接)
console.log(1 + false + undefined + [] + null + true + '珠峰培训' + [12] + 1);
/*
1 + false -> 1
1 + undefined -> NaN
*** NaN + [] -> 'NaN' ***
'NaN' + null -> 'NaNnull'
...字符串拼接
*/
/*
* 不论 i++ 还是 ++i,都是在自身基础上累加1的数学运算
* => 和 i+=1 或者 i=i+1 还不是完全一致的
* => i++是先运算,后自身累加1,而++i是先自身累加1,然后再去运算的(不论是否加了小括号)
*/
let i=1;
console.log(2-(i++)+(--i)+2+(++i)-(i--)-2); console.log(i);
/*
2-(i++) => 2-i / i++ => 1 i=2
1+(--i) => --i / 1+累减结果 => i=1 2
2+2 => 4
4+(++i) => ++i / 4+累加结果 => i=2 6
6-(i--) => 6-i / i-- => 4 i=1
4-2 => 2
i=1
*/
//let n="10";
//n++ => 11
//n+=1 => "101"
//n=n+1 => "101"
~~~

#### 9.
“1” “0”
~~~javascript
//=>alert输出的结构都是转换为字符串的
for (var i = 10; i >= 2; i--) {
if (i === 6) {
i-=2;
break;
} else if(i<=5) {
i=2;
} else {
i-=2;
continue;
}
i--;
alert(i);
}
alert(i);
~~~
#### 10.
~~~javascript
Number(""); //=>0
parseFloat(""); //=>NaN
!!"parseInt(NaN)"; //=>把非空字符串转化为布尔 true
!typeof typeof typeof [12,23]; //=>false
/*
typeof typeof typeof [12,23] => "string"
!"string" => false
*/
parseFloat("1.6px") + parseInt("1.2px");
/*
parseFloat("1.6px") => 1.6
parseInt("1.2px") => 1
1.6 + 1 => 2.6
*/
typeof "parseInt(undefined)" + 12 + !!Number('');
/*
typeof "parseInt(undefined)" => "string"
!!Number('') => false
"string" + 12 + false => "string12false"
*/
typeof !!parseInt(undefined) + !parseFloat(null);
/*
typeof !!parseInt(undefined)
parseInt(undefined) => parseInt("undefined") => NaN
!!NaN => false
typeof false => "boolean"
!parseFloat(null)
parseFloat(null) => NaN
!NaN => true
"boolean" + true => "booleantrue"
*/
~~~
- 0001.开课说明
- 0002.ECMAScript的发展历程
- 0003.WEB2.0时代-服务器端渲染,前后端不分离
- 0004.WEB2.0时代-前后端分离模式
- 0005.大前端时代概述
- 0006.前端需要的技术栈和学习技巧
- 0007.浏览器
- 0008.JS的三部分组成
- 0009.JS中创建变量的6种形式
- 0010.JS中变量的命名规范
- 0011.JS中的数据类型分类
- 0012.JS中常用的几种输出方式
- 0013.number属性类型详细解读1
- 0014.number数据类型详细解读2
- 0015.string数据类型详细解读1
- 0016.string数据类型详细解读2
- 0017.boolean数据类型详细解读
- 0018.object数据类型详细解读1
- 0019.object数据类型详细解读2
- 0020.谈谈学习
- 0021.数据类型检测
- 0022.浏览器底层渲染机制(堆栈内存和数据类型区别)
- 0023.关于数据类型区别的面试题
- 0024.课后作业讲解:数据类型转换
- 0025.课后作业讲解:堆栈内存处理
- 0026.课后作业讲解:阿里的一道经典面试题
- 0027.JS中三种常用的判断语句
- 0028.小实战:开关灯特效
- 0029.FOR循环和FOR IN循环
- 0030.课后作业讲解:关于循环判断和数据转化
- 0031.课后作业讲解:关于DOM对象的深入理解
- 0032.关于元素集合的相关操作(奇偶行变色)
- 0033.课后作业讲解:逻辑思维判断题
- reset.min.css
- 0034.(复习)前四天内容的综合复习梳理
- 0035.初窥函数:函数的作用、语法、形参
- 0036-0038.选项卡案例
- 0039.隔行变色案例:进一步强化自定义属性编程思想
- 0040.其它作业题的讲解(自定义属性强化)
- 0041.函数创建和执行的堆栈运行机制
- 0042.函数中的形参和实参
- 0043.函数中的实参集合ARGUMENTS
- 0044.函数中的返回值RETURN
- 0045.箭头函数和匿名函数
- 0046.两个等于比较时候的数据类型转换规则
- 0047.数组的基础结构和常规操作
- 0048.数组常用方法:增删改的五个方法
- 0049.数组常用方法:查询、拼接、转换为字符串
- 0050.数组常用方法:检测是否包含、排序和迭代
- 0051.数组去重:双FOR循环(数组塌陷和SPLICE删除优化)
- 0052.数组去重:对象键值对方式(ES6中SET)
- 0053.Math数学函数对象中常用的方法
- 0054.String字符串中常用的方法
- 0055.实战案例:时间字符串格式化
- 0056.实战案例:queryURLParams1
- 0057.实战案例:queryURLParams2
- 0058.实战案例:获取四位不重复的验证码
- 0059.阶段作业题讲解1(基础知识)
- 0060.阶段作业题讲解2(实战案例)
- 0061-0062.DOM操作中相关知识的复习
- 0063.DOM中的节点操作1
- 0064.DOM中的节点操作2
- utils
- 65.关于DOM的增删改