**#类原生代码:**
```
namespace php8;
class Top{
public function getTop(){
echo 'my name is getTop';
}
}
trait My{
public function getMy(){
echo 'my name is getMy';
}
}
/**
* @host www.yzmedu.com
*/
class Person extends Top{
public static $sex='nan';
public $name;
public $age;
const HOST='www.yzmedu.com';
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
public function say(){
echo "my name is {$this->name},my age is {$this->age}";
}
public static function show(){
echo "my name is php8";
}
use My;
}
```
 
**1.获取类的构造函数**
```
class Person{
public $name;
public $age;
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
}
$rf=new ReflectionClass('Person');
echo "<pre>";
print_r($rf->getConstructor());
echo "</pre>";
```
 
**2.获取类名**
```
class Person{}
$rf=new ReflectionClass('Person');
echo $rf->getName();
```
 
**3.获取类名的短名**
```
namespace php8;
class Person{
public $name;
public $age;
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
}
$rf=new \ReflectionClass('php8\Person');
echo $rf->getShortName();
```
**4.获取属性**
```
<?php
namespace php8;
class Person{
public $name;
public $age;
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
}
$rf=new \ReflectionClass('php8\Person');
$arr=$rf->getProperties();
echo "<pre>";
print_r($arr);
echo "</pre>";
?>
```
**5.获取方法**
```
namespace php8;
class Person{
public $name;
public $age;
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
public function say(){
echo "my name is {$this->name},my age is {$this->age}";
}
public static function show(){
echo "my name is php8";
}
}
$rf=new \ReflectionClass('php8\Person');
$arr=$rf->getMethods();
echo "<pre>";
print_r($arr);
echo "</pre>";
```
 
**6.获取命名空间**
```
<?php
namespace php8;
class Person{
public $name;
public $age;
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
public function say(){
echo "my name is {$this->name},my age is {$this->age}";
}
public static function show(){
echo "my name is php8";
}
}
$rf=new \ReflectionClass('php8\Person');
echo $rf->getNamespaceName();
?>
```
 
**7.获取父类**
```
<?php
namespace php8;
class Top{}
class Person extends Top{
public $name;
public $age;
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
public function say(){
echo "my name is {$this->name},my age is {$this->age}";
}
public static function show(){
echo "my name is php8";
}
}
$rf=new \ReflectionClass('php8\Person');
echo "<pre>";
print_r($rf->getParentClass());
echo "</pre>";
?>
```
 
**8.获取接口**
```
<?php
interface A{}
interface B{}
class C implements A,B{}
$rf=new \ReflectionClass('C');
echo "<pre>";
print_r($rf->getInterfaces());
echo "</pre>";
?>
```
 
**9.获取静态属性**
```
<?php
class Person{
public $name;
public $age;
static public $sex='nan';
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
public function say(){
echo "my name is {$this->name},my age is {$this->age}";
}
public static function show(){
echo "my name is php8";
}
}
$rf=new ReflectionClass('Person');
echo "<pre>";
print_r($rf->getStaticProperties());
echo "</pre>";
?>
```
 
**10.获取traits**
```
<?php
trait mytrait{
public function gettrait(){
echo "my name gettrait";
}
}
trait trait2{
public $job;
}
class Person{
public $name;
public $age;
static public $sex='nan';
const HOST='www.yzmedu.com';
use trait2;
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
public function say(){
echo "my name is {$this->name},my age is {$this->age}";
}
public static function show(){
echo "my name is php8";
}
use mytrait;
}
$rf=new ReflectionClass('Person');
echo "<pre>";
print_r($rf->getTraits());
echo "</pre>";
?>
```
 
**11.获取常量**
```
<?php
class Person{
public $name;
public $age;
static public $sex='nan';
const HOST='www.yzmedu.com';
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
public function say(){
echo "my name is {$this->name},my age is {$this->age}";
}
public static function show(){
echo "my name is php8";
}
}
$rf=new ReflectionClass('Person');
echo "<pre>";
print_r($rf->getConstants());
echo "</pre>";
?>
```
 
**12.获取文档注释**
```
<?php
/**
* @host www.yzmedu.com
*/
class Person{
public $name;
public $age;
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
public function say(){
echo "my name is {$this->name},my age is {$this->age}";
}
public static function show(){
echo "my name is php8";
}
}
$rf=new ReflectionClass('Person');
echo "<pre>";
print_r($rf->getDocComment());
echo "</pre>";
?>
```
 
**13.检查常量是否已经定义**
```
<?php
class Person{
public $name;
public $age;
const HOST='www.yzmedu.com';
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
public function say(){
echo "my name is {$this->name},my age is {$this->age}";
}
public static function show(){
echo "my name is php8";
}
}
$rf=new ReflectionClass('Person');
var_dump($rf->hasConstant('HOST'))
?>
```
 
**14.检查方法是否已定义**
```
<?php
class Person{
public $name;
public $age;
const HOST='www.yzmedu.com';
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
public function say(){
echo "my name is {$this->name},my age is {$this->age}";
}
public static function show(){
echo "my name is php8";
}
}
$rf=new ReflectionClass('Person');
var_dump($rf->hasMethod('show'))
?>
```
**15.检查属性是否已定义**
```
<?php
class Person{
public $name;
public $age;
const HOST='www.yzmedu.com';
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
public function say(){
echo "my name is {$this->name},my age is {$this->age}";
}
public static function show(){
echo "my name is php8";
}
}
$rf=new ReflectionClass('Person');
var_dump($rf->hasProperty('name'))
?>
```
 
**16.检查类是否是抽象类**
```
<?php
abstract class Person{
abstract function show();
}
$rf=new ReflectionClass('Person');
var_dump($rf->isAbstract())
?>
```
 
**17.检查类是否声明为final**
```
<?php
final class Person{
public $name;
public $age;
const HOST='www.yzmedu.com';
public function __construct($n,$a){
$this->name=$n;
$this->age=$a;
}
public function say(){
echo "my name is {$this->name},my age is {$this->age}";
}
public static function show(){
echo "my name is php8";
}
}
$rf=new ReflectionClass('Person');
var_dump($rf->isFinal())
?>
```
 
### **系统的学习PHP**
关注:PHP自学中心,回复相应的关键词,领取以下视频教程
**全面讲解正则表达式【php版】**
公众号里回复:763641
 
#### **还有其他的教程的关键词,请关注公众号查看每天分享的文章教程的头部**
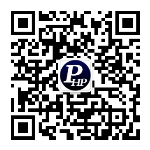
- 第1章:LNP Web环境搭建
- 1-1 Nginx1.19源码编译安装
- 1-2 Nginx1.19环境配置
- 1-3 Nginx1.19性能优化与测试
- 1-4 PHP8.0源码编译安装
- 1-5 PHP8.0环境配置
- 1-6 PHP8.0性能优化与测试
- 第2章:JIT即时编译
- 2-1 JIT编译原理
- 2-2 Tracing JIT和Function JIT编译引擎
- 2-3 Opcodes编译原理
- 2-4 Opcache和JIT功能开启
- 2-5 JIT高性能测试
- 第3章:PHP8的主要新特性
- 3-1 php8的命名参数
- 3-2 Reflection反射
- 3-3 注解
- 3-4 构造器属性提升
- 3-5 联合类型
- 3-6 Nullsafe空安全运算符
- 3-7 Match表达式
- 第4章:PHP8的新功能和类
- 4-1 PhpToken类
- 4-2 Stringable接口
- 4-3 WeakMap类
- 4-4 Str_contains函数
- 4-5 Str_starts_with和Str_ends_with函数
- 4-6 Fdiv函数
- 4-7 Get_resource_id函数
- 4-8 Get_debug_type函数
- 第5章:类型系统改进
- 5-1 新的Mixed伪类型
- 5-2 Static类方法的返回类型
- 第6章:错误处理方面的改进
- 6-1 系统函数引发TypeError和ValueError异常
- 6-2 Throw表达式抛出异常
- 6-3 无变量捕获的Catch
- 6-4 默认错误报告设置为E_ALL
- 6-5 默认情况下显示PHP启动错误
- 6-6 Assert断言默认情况下引发异常
- 6-7 操作符@不再抑制Fatal错误
- 6-8 PDO默认错误模式为ERRMODE_EXCEPTION
- 第7章:资源到对象的迁移
- 7-1 GdImage类对象替换了GD映像资源
- 7-2 CurlHandle类对象替换Curl处理程序
- 7-3 套接字扩展资源Socket是类对象
- 7-4 XMLWriter对象替换xmlwriter资源
- 第8章:PHP面向对象的编程更改
- 8-1 不兼容的方法签名的致命错误
- 8-2 严格执行类魔术方法签名
- 8-3 静态调用非静态类方法会导致致命错误
- 8-4 继承规则不适用于Private类方法
- 8-5 对象支持Class魔术常量
- 第9章:与字符串相关的更改
- 9-1 Substr和Iconv_substr偏移越境返回空字符串
- 9-2 加减运算符优先级高于点连接符
- 第10章:其他功能与特性
- 10-1 Printf采用新精度和宽度修饰符
- 10-2 内置Web服务器支持动态端口选择
- 10-3 参数列表和闭包Use列表中允许结尾逗号
- 10-4 隐式负数组键增量不会跳过负数
- 10-5 Crypt函数Salt为必选参数
- 10-6 调用禁用函数或类为未定义状态
- 10-7 可选参数之后禁止出现必选参数
- 第11章:弃用的函数与方法
- 11-1 ReflectionFunction::isDisabled弃用
- 11-2 ReflectionParameter::getClass弃用
- 11-3 ReflectionParameter::isArray弃用
- 11-4 ReflectionParameter::isCallable弃用
- 11-5 ReflectionClass::export弃用
- 11-6 ReflectionFunction::export弃用
- 11-7 Get_defined_functions改进禁用函数
- 11-8 24个PostgreSQL的别名函数弃用